Data teams across organizations face a critical challenge: while AI adoption has exploded, most implementations remain trapped in basic chatbot functionality, missing the sophisticated orchestration capabilities that drive real business transformation. Engineering teams waste countless hours rebuilding similar AI functionality across projects, struggling with context management failures, integration complexity, and operational fragility that breaks with every library update. Organizations that successfully implement comprehensive AI orchestration frameworks using LangChain report deployment cycles that are 3-5× faster and manual data engineering burdens reduced by 60-80%.
LangChain has emerged as the leading framework for building sophisticated AI applications, offering a comprehensive ecosystem that transforms how developers approach everything from document analysis to complex data integration workflows. With its modular architecture comprising langchain-core, domain-specific modules, and partner integrations, LangChain enables seamless customization of chains with built-in streaming and observability capabilities.
This article explores the most impactful LangChain use cases, from foundational applications like summarization and chatbots to cutting-edge implementations such as multi-agent systems and real-time data processing. You'll discover practical implementations, learn advanced techniques, and understand how to build production-ready AI applications that solve real business problems.
What Do You Need to Know Before Starting with LangChain?
Before exploring LangChain use cases, make sure your data is easy to access. It often lives in multiple sources, making it hard to use for training. No-code data-movement platforms like Airbyte can streamline data integration.

Airbyte offers 600+ pre-built data connectors plus:
Below is a quick example that uses PyAirbyte to read a CSV file from Google Drive and convert it into a list of LangChain-ready Document
objects (replace the placeholders with your own values):
%pip install --quiet airbyte
import airbyte as ab
service_json = ab.get_secret('service_json')
source = ab.get_source(
"source-google-drive",
install_if_missing=True,
config={
"folder_url": "https://drive.google.com/drive/folders/xxxxxxxxxxxxxxxx",
"credentials": {
"auth_type": "Service",
"service_account_info": f"""{service_json}""",
},
"streams": [{
"name": "NFLX",
"globs": ["**/*.csv"],
"format": {"filetype": "csv"},
"validation_policy": "Emit Record",
"days_to_sync_if_history_is_full": 3
}]
},
)
source.check() # verify connection
source.select_all_streams()
read_result = source.read()
documents_list = []
for key, value in read_result.items():
docs = value.to_documents()
documents_list.extend(docs)
print(documents_list[0]) # inspect one row
You can now chunk these documents, embed them, and load them into a vector database for RAG pipelines. The integration supports incremental updates and automatic deduplication, reducing embedding costs while maintaining data freshness. The platform's direct loading feature reduces compute costs by 50-70% when syncing to BigQuery and Snowflake, accelerating LangChain workflows requiring fresh data. (See the full tutorial.)
How Can You Use LangChain for Document Summarization?
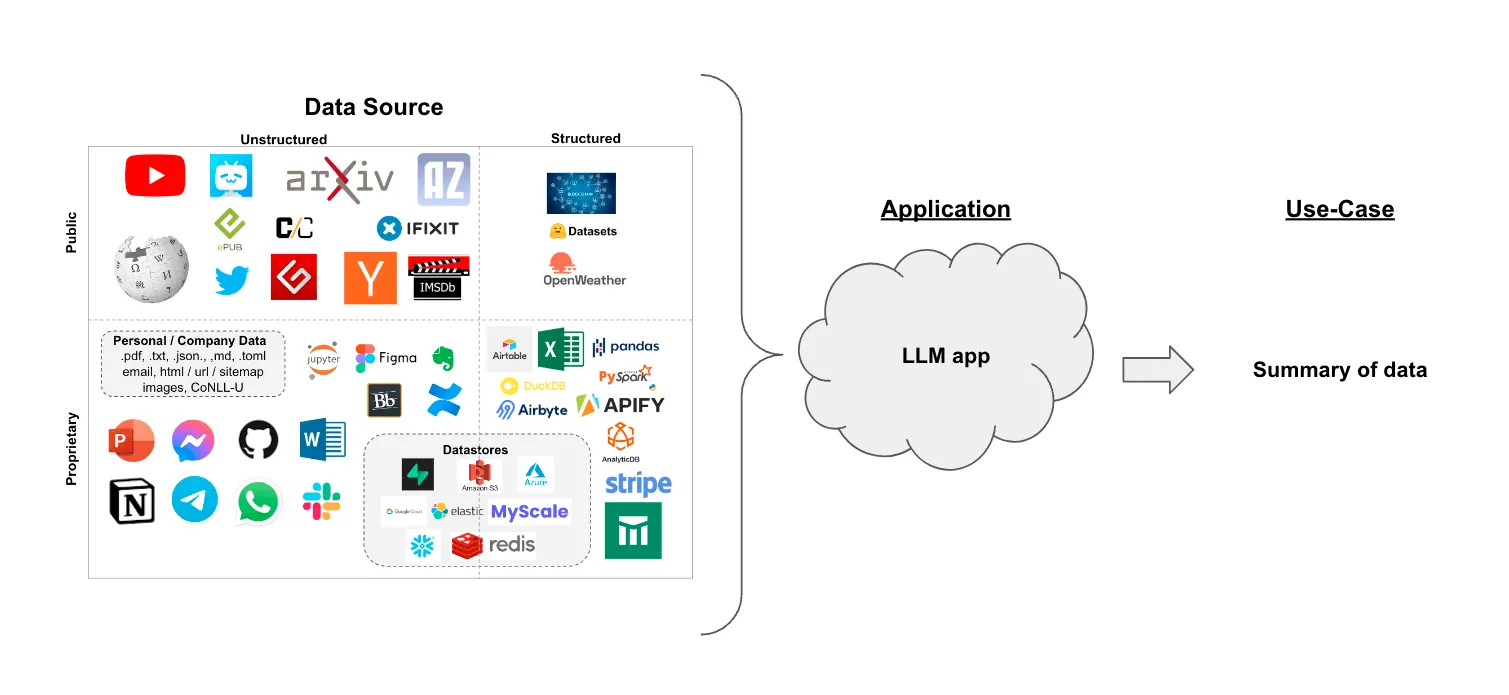
Summarization helps you condense content such as articles, chat logs, legal documents, and research papers. Because LLMs have context-length limits, larger texts must be split into chunks and then summarized with approaches like stuff (put everything in one prompt) or map-reduce. Modern implementations leverage advanced chunking strategies and context-optimization techniques to improve accuracy while reducing token usage.
Prerequisites
%pip install --upgrade --quiet langchain-openai langchain python-dotenv
from dotenv import load_dotenv
import os
load_dotenv()
openai_api_key = os.getenv('OPENAI_API_KEY', 'YOUR_API_KEY')
Summarizing Short Text
from langchain_openai import OpenAI
from langchain.prompts import PromptTemplate
from langchain.chains import LLMChain
llm = OpenAI(
temperature=0,
model_name='gpt-3.5-turbo-instruct',
openai_api_key=openai_api_key
)
template = """
%INSTRUCTIONS:
Please summarize the following piece of text.
Respond in a manner that a 5-year-old would understand.
Focus on the main ideas and key points.
%TEXT:
{text}
"""
prompt = PromptTemplate(input_variables=["text"], template=template)
chain = LLMChain(llm=llm, prompt=prompt)
confusing_text = """
For the next 130 years, debate raged over the fundamental nature of light...
"""
result = chain.run(text=confusing_text)
print(result)
Summarizing Longer Text with Advanced Techniques
from langchain.chains.summarize import load_summarize_chain
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain.schema import Document
# Load text content
with open('data/PaulGrahamEssays/good.txt') as f:
text = f.read()
# Advanced text splitting with semantic awareness
text_splitter = RecursiveCharacterTextSplitter(
separators=["\n\n", "\n", ".", "!", "?"],
chunk_size=4000,
chunk_overlap=200,
length_function=len
)
docs = text_splitter.create_documents([text])
# Use map-reduce chain for better handling of long documents
chain = load_summarize_chain(
llm,
chain_type='map_reduce',
verbose=True,
map_prompt=PromptTemplate.from_template(
"Summarize the following text, focusing on key insights:\n\n{text}"
),
combine_prompt=PromptTemplate.from_template(
"Combine these summaries into a comprehensive overview:\n\n{text}"
)
)
summary = chain.run(docs)
print(summary)
Context-Aware Summarization
Advanced summarization implementations use custom prompt templates that adapt to document types and include metadata for better context understanding. This approach reduces hallucination while maintaining accuracy across different content formats. Healthcare organizations have successfully implemented LangChain summarization for clinical notes, reducing documentation time from 30 minutes to 3 minutes while maintaining accuracy through multi-layer validation systems. Legal firms leverage LangChain's document loader integrations to process contracts and case documents through specialized summarization chains that preserve critical terminology and regulatory references.
How Do You Build Conversational Agents with LangChain?
LangChain makes it easy to build conversational agents that incorporate memory and context persistence. Modern chatbot implementations leverage streaming responses, conversation memory, and multi-turn dialogue management for more natural interactions. Leading implementations now handle millions of conversations monthly while maintaining context across complex multi-step interactions.
Basic Chatbot with Memory
from langchain_openai import OpenAI
from langchain.chains import ConversationChain
from langchain.memory import ConversationBufferMemory
from langchain.prompts import PromptTemplate
llm = OpenAI(
temperature=0.5,
model_name="gpt-3.5-turbo-instruct",
openai_api_key=openai_api_key
)
prompt = PromptTemplate.from_template("""
You are a helpful assistant that provides clear, accurate information.
Your responses should be conversational and engaging.
Current conversation:
{history}
User: {input}
Assistant:
""")
memory = ConversationBufferMemory(memory_key="history")
chat_chain = ConversationChain(llm=llm, memory=memory, prompt=prompt, verbose=True)
chat_chain.predict(input="Hi there!")
chat_chain.predict(input="Can you explain what LangChain is?")
The ConversationBufferMemory
object stores the dialogue history and feeds it back into each prompt, enabling multi-turn context without manual engineering. You can easily swap this for ConversationSummaryMemory
, VectorStoreRetrieverMemory
, or custom memory modules for more sophisticated use cases such as summarizing long chats or retrieving domain-specific context.
Advanced Agent Architecture with Tools
from langchain.agents import initialize_agent, Tool
from langchain.agents import AgentType
from langchain.tools import DuckDuckGoSearchRun
from langchain.memory import ConversationSummaryBufferMemory
# Initialize tools
search = DuckDuckGoSearchRun()
tools = [
Tool(
name="Search",
func=search.run,
description="Search for current information and facts"
)
]
# Enhanced memory management
memory = ConversationSummaryBufferMemory(
llm=llm,
max_token_limit=4000,
memory_key="chat_history",
return_messages=True
)
agent = initialize_agent(
tools,
llm,
agent=AgentType.CONVERSATIONAL_REACT_DESCRIPTION,
verbose=True,
memory=memory,
max_iterations=3,
early_stopping_method="generate"
)
response = agent.run("What are the latest developments in AI agents?")
print(response)
Enterprise chatbot implementations combine LangChain's conversational capabilities with specialized tools and memory systems. Customer service organizations deploy chatbots using ConversationBufferMemory
for session-based interactions and ConversationSummaryMemory
for long-term customer relationship tracking. Financial institutions implement compliance-aware chatbots that maintain audit trails while providing personalized assistance through integration with CRM systems and regulatory databases.
What Are Advanced Observability and Monitoring Techniques for LangChain Applications?
The emergence of specialized observability platforms represents a fundamental shift in managing LLM application lifecycles. LangChain's inherent complexity with nested chains, agentic workflows, and external tool integrations creates unique monitoring challenges that traditional APM tools cannot address. The non-deterministic nature of generative models further complicates performance isolation, requiring specialized tracing capabilities that map token consumption patterns, context propagation across chains, and third-party API latency hotspots.
LangSmith Integration for Production Monitoring
from langchain.smith import RunEvalConfig, run_on_dataset
from langchain.callbacks.tracers import LangChainTracer
from langchain.callbacks.manager import CallbackManager
# Initialize LangSmith tracing
tracer = LangChainTracer(
project_name="production-agent",
tags=["version:1.2", "environment:prod"]
)
callback_manager = CallbackManager([tracer])
# Enhanced chain with observability
chain = ConversationChain(
llm=llm,
memory=memory,
callback_manager=callback_manager,
verbose=True
)
# Run with automatic tracing
result = chain.predict(input="Analyze quarterly sales data")
Cost Intelligence and Performance Optimization
from langchain.callbacks import get_openai_callback
from langchain.cache import SQLiteCache
from langchain.globals import set_llm_cache
# Enable semantic caching
set_llm_cache(SQLiteCache(database_path=".langchain.db"))
# Track token usage
with get_openai_callback() as cb:
result = chain.predict(input="Generate monthly report")
print(f"Total Tokens: {cb.total_tokens}")
print(f"Total Cost: ${cb.total_cost:.4f}")
Enterprise-Grade Deployment Monitoring
For large-scale deployments, OpenTelemetry integration provides distributed tracing across hybrid infrastructures, Kubernetes-native deployment monitoring, and data redaction for compliance requirements. Financial services organizations leverage these capabilities to maintain audit trails for regulatory requirements while optimizing AI application performance across distributed environments. LangSmith's evaluation suites enable continuous quality assessment through automated testing pipelines that validate model outputs against business-specific criteria, ensuring production reliability while tracking performance degradation over time.
How Do RAG-Enhanced Agent Systems Transform Enterprise Applications?
RAG-enhanced agents have evolved from supplementary techniques to core architectural paradigms within LangChain applications. Modern implementations move beyond simple retrieval to context-aware reasoning systems that combine iterative refinement of retrieved context through hypothesis-driven retrieval, evidence synthesis, and self-correction mechanisms.
Multi-Stage Reasoning Implementation
from langchain.agents import AgentExecutor, create_react_agent
from langchain.tools.retriever import create_retriever_tool
from langchain.vectorstores import Chroma
from langchain.embeddings import OpenAIEmbeddings
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain.prompts import PromptTemplate
# Create vector store from documents
text_splitter = RecursiveCharacterTextSplitter(
chunk_size=1000,
chunk_overlap=200
)
docs = text_splitter.split_documents(documents_list)
vectorstore = Chroma.from_documents(
docs,
OpenAIEmbeddings(),
persist_directory="./chroma_db"
)
# Create retrieval tool
retriever_tool = create_retriever_tool(
vectorstore.as_retriever(search_kwargs={"k": 5}),
"knowledge_base",
"Search for information in the company knowledge base"
)
# Enhanced agent prompt for RAG
prompt = PromptTemplate.from_template("""
You are a knowledgeable assistant with access to a comprehensive knowledge base.
When answering questions, first retrieve relevant information, then synthesize
a comprehensive response based on the retrieved context.
Available tools:
{tools}
Question: {input}
{agent_scratchpad}
""")
agent = create_react_agent(llm, [retriever_tool], prompt)
agent_executor = AgentExecutor(agent=agent, tools=[retriever_tool], verbose=True)
response = agent_executor.invoke({
"input": "What are the key technical considerations for data integration?"
})
Hybrid Tool Integration Architecture
from langchain.tools import SQLDatabaseTool
from langchain.utilities import SQLDatabase
from langchain.agents.agent_toolkits import SQLDatabaseToolkit
# Initialize database connection
db = SQLDatabase.from_uri("sqlite:///sales_data.db")
toolkit = SQLDatabaseToolkit(db=db, llm=llm)
# Combine RAG and SQL tools
combined_tools = [retriever_tool] + toolkit.get_tools()
# Create hybrid agent
hybrid_agent = create_react_agent(llm, combined_tools, prompt)
hybrid_executor = AgentExecutor(
agent=hybrid_agent,
tools=combined_tools,
verbose=True,
max_iterations=5
)
# Query combining knowledge base and database
response = hybrid_executor.invoke({
"input": "Analyze Q3 sales performance and provide strategic recommendations"
})
Enterprise RAG implementations leverage LangChain's document loader ecosystem, which now supports over 230 data sources including Slack, Notion, and SAP systems. Organizations achieve context-aware reasoning through hybrid retrieval architectures that combine vector similarity search with keyword-based retrieval, optimizing context precision while reducing hallucination rates. Legal firms deploy RAG systems using ParentDocumentRetriever
to maintain document hierarchy context, enabling accurate citation tracking across complex regulatory documents.
What Are the Key Techniques for Multi-Agent Orchestration in LangChain?
Multi-agent systems represent one of the most sophisticated applications of LangChain, enabling complex workflows through coordinated agent interactions. The introduction of LangGraph revolutionized agent architecture by enabling explicit state transitions through graph-based workflows, supporting cyclical operations and consensus mechanisms in distributed tasks.
LangGraph Architecture for Stateful Workflows
from langgraph.graph import StateGraph, END
from langgraph.prebuilt import create_react_agent
from typing import TypedDict, List
class AgentState(TypedDict):
messages: List[str]
data: dict
next_action: str
def research_agent(state: AgentState):
"""Agent responsible for data research and analysis"""
messages = state["messages"]
research_results = {"findings": "Market analysis complete"}
return {
"messages": messages + ["Research completed"],
"data": research_results,
"next_action": "review"
}
def review_agent(state: AgentState):
"""Agent responsible for reviewing and validating results"""
messages = state["messages"]
data = state["data"]
return {
"messages": messages + ["Review completed"],
"data": data,
"next_action": "finalize"
}
def should_continue(state: AgentState):
return state["next_action"]
workflow = StateGraph(AgentState)
workflow.add_node("research", research_agent)
workflow.add_node("review", review_agent)
workflow.add_conditional_edges(
"research",
should_continue,
{"review": "review", "finalize": END}
)
workflow.add_edge("review", END)
workflow.set_entry_point("research")
app = workflow.compile()
initial_state = {
"messages": ["Starting analysis"],
"data": {},
"next_action": "research"
}
result = app.invoke(initial_state)
print(result)
Hierarchical Agent Systems
from langchain.agents import AgentExecutor
from langchain.schema import HumanMessage
class SupervisorAgent:
def __init__(self, sub_agents):
self.sub_agents = sub_agents
self.llm = llm
def route_task(self, task):
routing_prompt = f"""
Analyze this task and determine which agent should handle it:
Task: {task}
Available agents:
- data_agent: Handles data analysis and processing
- research_agent: Performs research and fact-checking
- reporting_agent: Creates reports and summaries
Return only the agent name.
"""
response = self.llm.invoke([HumanMessage(content=routing_prompt)])
return response.content.strip()
def execute_task(self, task):
agent_name = self.route_task(task)
if agent_name in self.sub_agents:
return self.sub_agents[agent_name].run(task)
else:
return "No suitable agent found"
supervisor = SupervisorAgent({
"data_agent": data_analysis_agent,
"research_agent": research_agent,
"reporting_agent": reporting_agent
})
result = supervisor.execute_task("Analyze customer churn patterns")
LangGraph's deferred nodes enable asynchronous workflow execution, allowing multi-agent systems to handle long-running processes without blocking operations. Enterprise implementations leverage checkpointing mechanisms to maintain state persistence across distributed agent collaborations, ensuring workflow continuity during system failures. Financial services organizations deploy multi-agent systems for fraud detection, combining transaction analysis agents with risk assessment agents and compliance validation agents through LangGraph's stateful orchestration.
What Are Real-Time Data Integration Architectures for LangChain Applications?
Real-time data integration represents a critical advancement in LangChain applications, enabling immediate processing of streaming data through event-driven architectures. Modern implementations combine Apache Kafka streaming with LangChain's agentic workflows to create responsive systems that process data as it arrives, triggering intelligent actions based on real-time insights.
Event-Driven Pipeline Implementation
from langchain.agents import AgentExecutor
from langchain.callbacks.streaming_stdout import StreamingStdOutCallbackHandler
import asyncio
from typing import AsyncIterator
import kafka
class RealTimeIntegrationPipeline:
def __init__(self, kafka_config, agent_executor):
self.kafka_consumer = kafka.KafkaConsumer(
'data-events',
bootstrap_servers=kafka_config['servers'],
value_deserializer=lambda x: json.loads(x.decode('utf-8'))
)
self.agent = agent_executor
self.processing_queue = asyncio.Queue()
async def consume_events(self):
"""Consume real-time events from Kafka streams"""
for message in self.kafka_consumer:
event_data = message.value
await self.processing_queue.put(event_data)
async def process_stream(self):
"""Process streaming data with LangChain agents"""
while True:
try:
event_data = await self.processing_queue.get()
# Transform event data for LangChain processing
analysis_prompt = f"""
Analyze this real-time event and determine required actions:
Event Type: {event_data['type']}
Payload: {event_data['payload']}
Timestamp: {event_data['timestamp']}
Provide actionable insights and recommendations.
"""
result = await self.agent.ainvoke({
"input": analysis_prompt
})
# Trigger downstream actions based on analysis
await self.handle_analysis_result(result, event_data)
except Exception as e:
await self.handle_processing_error(e, event_data)
async def handle_analysis_result(self, result, original_event):
"""Handle processed results and trigger appropriate actions"""
if result.get('urgency') == 'high':
await self.trigger_alert(result, original_event)
elif result.get('action_required'):
await self.queue_action(result['recommended_action'])
Microservices Integration Architecture
from langchain.agents import initialize_agent, Tool
from langchain.schema import Document
import aiohttp
import asyncio
class MicroservicesOrchestrator:
def __init__(self, llm, service_endpoints):
self.llm = llm
self.service_endpoints = service_endpoints
self.tools = self.create_service_tools()
self.agent = initialize_agent(
self.tools,
llm,
agent=AgentType.ZERO_SHOT_REACT_DESCRIPTION,
verbose=True
)
def create_service_tools(self):
tools = []
for service_name, endpoint in self.service_endpoints.items():
tool = Tool(
name=f"{service_name}_service",
func=lambda query, svc=service_name: self.call_service(svc, query),
description=f"Call {service_name} microservice for specialized processing"
)
tools.append(tool)
return tools
async def call_service(self, service_name, query):
"""Make asynchronous calls to microservices"""
endpoint = self.service_endpoints[service_name]
async with aiohttp.ClientSession() as session:
async with session.post(
f"{endpoint}/process",
json={"query": query}
) as response:
result = await response.json()
return result
async def orchestrate_workflow(self, user_request):
"""Orchestrate complex workflows across microservices"""
workflow_plan = await self.agent.ainvoke({
"input": f"Plan workflow for: {user_request}"
})
return workflow_plan
Dynamic Decision-Making Systems
Real-time integration architectures enable dynamic decision-making through continuous data processing. LangChain agents monitor streaming data from IoT sensors, CRM updates, and transaction logs, triggering immediate responses based on pattern recognition and anomaly detection. Retail organizations implement real-time inventory-customer alignment systems that process purchase events through Kafka topics, generate personalized recommendations via LangChain agents, and update customer interfaces within milliseconds. These systems achieve end-to-end latency under 1.5 seconds while maintaining transactional consistency through LangGraph's checkpoint memory system.
How Can You Build Real-Time Data Processing Applications with LangChain?
Real-time data processing applications combine streaming data ingestion with AI-powered analysis and decision-making, enabling immediate responses to data changes while maintaining sophisticated reasoning capabilities.
Streaming Data Integration
from langchain.schema import Document
from langchain.callbacks.streaming_stdout import StreamingStdOutCallbackHandler
import asyncio
from typing import AsyncIterator
async def stream_processor(data_stream: AsyncIterator[dict]):
async for data_chunk in data_stream:
doc = Document(
page_content=data_chunk["content"],
metadata=data_chunk["metadata"]
)
streaming_llm = OpenAI(
streaming=True,
callbacks=[StreamingStdOutCallbackHandler()],
temperature=0.3
)
result = await streaming_llm.ainvoke(
f"Analyze this real-time data: {doc.page_content}"
)
yield result
async def main():
async def mock_data_stream():
for i in range(10):
yield {
"content": f"Real-time event {i}",
"metadata": {"timestamp": i, "source": "sensor"}
}
await asyncio.sleep(1)
async for result in stream_processor(mock_data_stream()):
print(f"Processed: {result}")
asyncio.run(main())
Event-Driven Architecture
from langchain.agents import AgentExecutor
from langchain.memory import ConversationBufferMemory
import json
class EventDrivenAgent:
def __init__(self, agent_executor):
self.agent = agent_executor
self.memory = ConversationBufferMemory()
async def process_event(self, event_data):
event_type = event_data.get("type")
payload = event_data.get("payload")
if event_type == "data_update":
response = await self.agent.ainvoke({
"input": f"Process data update: {payload}",
"chat_history": self.memory.chat_memory.messages
})
elif event_type == "alert":
response = await self.agent.ainvoke({
"input": f"Handle alert: {payload}",
"chat_history": self.memory.chat_memory.messages
})
self.memory.save_context(
{"input": json.dumps(event_data)},
{"output": response["output"]}
)
return response
Advanced streaming implementations leverage Apache NiFi's Kubernetes-native orchestration and stateless execution mode with transactional rollbacks. Google Cloud Dataflow's autoscaling innovations handle high-volume streaming data with minimal latency, enabling real-time LangChain applications that process IoT feeds and social media streams. These architectures support exactly-once semantics through Kafka Connect, guaranteeing data integrity for mission-critical applications in financial and healthcare sectors.
What Are AI-Powered Data Quality Enforcement Techniques in LangChain?
AI-powered data quality enforcement represents a paradigm shift in how LangChain applications manage data governance. Machine learning-driven integration reduces data mapping errors while accelerating pipeline development through automated schema mapping, self-correcting data contracts, and proactive anomaly detection using LLM-generated quality rules.
Automated Schema Mapping and Reconciliation
from langchain.agents import AgentExecutor, create_react_agent
from langchain.tools import StructuredTool
from langchain.prompts import PromptTemplate
import json
from typing import Dict, List
class SchemaMapperAgent:
def __init__(self, llm):
self.llm = llm
self.mapping_history = {}
def analyze_schema_tool(self, schema_json: str) -> str:
"""Analyze schema structure and suggest mappings"""
schema = json.loads(schema_json)
analysis_prompt = f"""
Analyze this schema and identify:
1. Primary data types and structures
2. Potential mapping conflicts with standard formats
3. Recommended transformations for integration
Schema: {schema}
"""
response = self.llm.invoke(analysis_prompt)
return response
def create_mapping_rules(self, source_schema: Dict, target_schema: Dict) -> Dict:
"""Generate intelligent mapping rules between schemas"""
mapping_prompt = f"""
Create mapping rules between these schemas:
Source: {source_schema}
Target: {target_schema}
Consider:
- Field name similarities using NLP matching
- Data type compatibility and conversion needs
- Nested structure flattening or expansion
- Missing field handling strategies
Return mapping rules in JSON format.
"""
response = self.llm.invoke(mapping_prompt)
return json.loads(response)
schema_tool = StructuredTool.from_function(
func=SchemaMapperAgent(llm).analyze_schema_tool,
name="schema_analyzer",
description="Analyze schema structure and suggest intelligent mappings"
)
schema_agent = create_react_agent(llm, [schema_tool], PromptTemplate.from_template("""
Analyze the provided schema and generate mapping recommendations.
Available tools: {tools}
Schema to analyze: {input}
{agent_scratchpad}
"""))
schema_executor = AgentExecutor(
agent=schema_agent,
tools=[schema_tool],
verbose=True
)
Self-Correcting Data Contracts
from langchain.schema import BaseOutputParser
from langchain.prompts import PromptTemplate
import re
from typing import Any
class DataContractValidator:
def __init__(self, llm):
self.llm = llm
self.validation_rules = {}
def generate_quality_rules(self, data_sample: Dict) -> List[str]:
"""Generate data quality rules using LLM analysis"""
rule_generation_prompt = f"""
Analyze this data sample and generate quality validation rules:
{data_sample}
Generate rules for:
1. Data type validation
2. Value range constraints
3. Format compliance checks
4. Referential integrity rules
5. Freshness and completeness requirements
Return as executable Python validation functions.
"""
rules = self.llm.invoke(rule_generation_prompt)
return self.parse_validation_rules(rules)
def dynamic_schema_adaptation(self, schema_drift_event: Dict):
"""Adapt to schema changes using AI reasoning"""
adaptation_prompt = f"""
Schema drift detected: {schema_drift_event}
Determine:
1. Impact assessment on existing pipelines
2. Required transformations to maintain compatibility
3. Rollback procedures if adaptation fails
4. Updated validation rules for new schema
Generate adaptation plan with migration steps.
"""
adaptation_plan = self.llm.invoke(adaptation_prompt)
return self.execute_adaptation_plan(adaptation_plan)
def validate_with_context(self, data: Dict, metadata: Dict) -> Dict:
"""Validate data considering contextual information"""
context_prompt = f"""
Validate this data considering its context:
Data: {data}
Metadata: {metadata}
Check for:
- Contextual anomalies (unusual patterns for this data source)
- Cross-field dependencies and consistency
- Temporal consistency with historical patterns
- Business rule compliance based on metadata
Return validation results with confidence scores.
"""
validation_result = self.llm.invoke(context_prompt)
return json.loads(validation_result)
data_validator = DataContractValidator(llm)
Proactive Anomaly Detection and Remediation
from langchain.agents import initialize_agent, Tool
from langchain.memory import ConversationSummaryMemory
import numpy as np
from datetime import datetime
class AnomalyDetectionAgent:
def __init__(self, llm):
self.llm = llm
self.anomaly_patterns = {}
self.remediation_history = []
def pattern_analysis_tool(self, data_stream: str) -> str:
"""Analyze data patterns for anomaly detection"""
analysis_prompt = f"""
Analyze this data stream for anomalies:
{data_stream}
Identify:
1. Statistical outliers using context-aware thresholds
2. Temporal pattern deviations from historical norms
3. Cross-field correlation breaks
4. Data completeness degradation signals
Provide anomaly confidence scores and root cause hypotheses.
"""
return self.llm.invoke(analysis_prompt)
def generate_remediation_workflow(self, anomaly_report: Dict) -> Dict:
"""Generate automated remediation workflows"""
remediation_prompt = f"""
Design remediation workflow for this anomaly:
{anomaly_report}
Create workflow including:
1. Immediate containment actions
2. Data correction procedures
3. Pipeline adjustment recommendations
4. Monitoring enhancements to prevent recurrence
Prioritize actions by business impact and implementation complexity.
"""
workflow = self.llm.invoke(remediation_prompt)
return self.parse_workflow_steps(workflow)
# Create anomaly detection tools
anomaly_tools = [
Tool(
name="pattern_analyzer",
func=AnomalyDetectionAgent(llm).pattern_analysis_tool,
description="Analyze data patterns for intelligent anomaly detection"
)
]
anomaly_agent = initialize_agent(
anomaly_tools,
llm,
agent=AgentType.CONVERSATIONAL_REACT_DESCRIPTION,
memory=ConversationSummaryMemory(llm=llm),
verbose=True
)
AI-powered data quality enforcement transforms traditional rule-based validation into adaptive, intelligent governance systems. LangChain's natural language processing capabilities interpret unstructured metadata, applying transformers to reconcile schema variations across data sources. Organizations achieve automated policy enforcement through LangChain agents that validate regulatory compliance during ingestion, while dynamic access control systems classify data sensitivity to enforce role-based access protocols. These implementations reduce data quality incidents and improve reliability scores through predictive quality monitoring and automatic remediation workflows.
What Are the Enterprise Integration Patterns for LangChain Deployment?
Enterprise deployment of LangChain applications requires sophisticated integration patterns that ensure scalability, security, and compliance across complex organizational environments.
Kubernetes-Native Deployment Architecture
from langchain.cache import RedisCache
from langchain.globals import set_llm_cache
import redis, os
from datetime import datetime
redis_client = redis.Redis(
host=os.getenv("REDIS_HOST", "localhost"),
port=int(os.getenv("REDIS_PORT", 6379)),
db=int(os.getenv("REDIS_DB", 0))
)
set_llm_cache(RedisCache(redis_client))
class ProductionChain:
def __init__(self):
self.llm = OpenAI(
temperature=0.3,
max_retries=3,
request_timeout=30
)
self.memory = ConversationBufferMemory()
async def process_request(self, request_data):
try:
if not self.validate_input(request_data):
raise ValueError("Invalid input data")
result = await self.llm.ainvoke(request_data["prompt"])
self.log_interaction(request_data, result)
return {
"success": True,
"result": result,
"timestamp": datetime.now().isoformat()
}
except Exception as e:
self.log_error(e, request_data)
return {
"success": False,
"error": str(e),
"timestamp": datetime.now().isoformat()
}
def validate_input(self, data):
required_fields = ["prompt", "user_id"]
return all(field in data for field in required_fields)
def log_interaction(self, request, response):
pass
def log_error(self, error, request_data):
pass
Security and Compliance Framework
from langchain.schema import BaseMessage
from cryptography.fernet import Fernet
import hashlib
class SecureChain:
def __init__(self, encryption_key):
self.fernet = Fernet(encryption_key)
self.llm = OpenAI()
def encrypt_data(self, data):
return self.fernet.encrypt(data.encode())
def decrypt_data(self, encrypted_data):
return self.fernet.decrypt(encrypted_data).decode()
def hash_pii(self, text):
return hashlib.sha256(text.encode()).hexdigest()
async def secure_process(self, encrypted_request):
request_data = self.decrypt_data(encrypted_request)
sanitized_data = self.sanitize_pii(request_data)
result = await self.llm.ainvoke(sanitized_data)
encrypted_response = self.encrypt_data(result)
return encrypted_response
def sanitize_pii(self, text):
return text
Monitoring and Observability Integration
from prometheus_client import Counter, Histogram, generate_latest
import time
REQUEST_COUNT = Counter('langchain_requests_total', 'Total requests')
REQUEST_DURATION = Histogram('langchain_request_duration_seconds', 'Request duration')
ERROR_COUNT = Counter('langchain_errors_total', 'Total errors')
class MonitoredChain:
def __init__(self):
self.llm = OpenAI()
@REQUEST_DURATION.time()
async def monitored_invoke(self, prompt):
REQUEST_COUNT.inc()
try:
start_time = time.time()
result = await self.llm.ainvoke(prompt)
duration = time.time() - start_time
self.record_success_metrics(duration, result)
return result
except Exception as e:
ERROR_COUNT.inc()
self.record_error_metrics(e)
raise
def record_success_metrics(self, duration, result):
pass
def record_error_metrics(self, error):
pass
def get_metrics(self):
return generate_latest()
Enterprise integration patterns leverage Terraform automation for infrastructure deployment, while Azure Data Factory integrations provide managed identity authentication for granular access control. Multi-data plane architectures keep sensitive data on-premises while synchronizing metadata to cloud LLMs, addressing data sovereignty requirements across regulatory jurisdictions. Organizations implement zero-copy cloning through Snowflake partnerships for development environments, reducing storage costs while maintaining production-quality testing capabilities.
FAQ: Building Production-Ready AI Applications with LangChain
What is LangChain used for?
LangChain is a modular framework for building advanced AI applications like document summarization, RAG pipelines, chatbots, multi-agent systems, and real-time data integration. It streamlines context management, orchestration, and observability for production-scale AI systems.
Why is LangChain better than basic chatbots?
Unlike basic chatbot frameworks, LangChain supports complex workflows like multi-step reasoning, tool integrations, vector database retrieval (RAG), and multi-agent collaboration. This enables real business applications beyond simple Q&A chatbots.
How can LangChain speed up AI development?
Organizations using LangChain report 3–5× faster deployment cycles and 60–80% reductions in manual data-engineering work. Its reusable chains, streaming capabilities, and integration with tools like Airbyte and LangSmith eliminate the need to rebuild common AI functions from scratch.
What are LangChain’s key enterprise features?
LangChain offers streaming responses, advanced memory management, observability via LangSmith, vector-store integrations, and hybrid agent architectures. These features help organizations build scalable, secure, and maintainable AI applications with full monitoring and cost tracking.
How does LangChain support real-time data and multi-agent systems?
LangChain integrates with event-driven architectures (e.g., Kafka streams) and vector databases for real-time processing. Tools like LangGraph enable stateful workflows and multi-agent orchestration, allowing enterprises to build dynamic, collaborative AI systems that handle complex tasks across distributed services.