Python & REST API: 4 Practical Use Cases for Data Engineer
Python is a robust programming language that offers numerous resources for a wide multitude of applications. Among these resources are libraries designed to create and manage REST APIs that enable you to streamline information sharing between different applications.
While there are multiple ways to create and interact with REST API in various programming languages, using Python is comparatively beneficial. It provides you the flexibility to perform complex operations almost effortlessly.
Through this article, you will learn about the REST APIs in Python, its benefits, the methods involved, use cases, and the necessary libraries to build your own REST API.
What Is REST API?
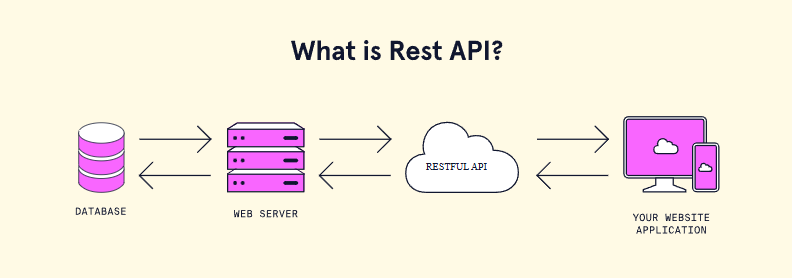
REST API, or Application Programming Interface, represents a set of rules and regulations to allow seamless communication between various applications. The REST—representational state transfer—component highlights a software architecture that defines the underlying working principles of the API. Some of the key principles of REST include:
- Uniform Interface: A uniform interface is crucial to establish communication between the client and the server, independent of the application. The server can transmit information in a format different from its internal representation in server-side applications.
- Independent Client-Server Connection: Decoupling the client-server connection is a necessary step to ensure the client interacts with the server through a Uniform Resource Identifier (URI). The server processes only the required information requests sent by the client.
- Layered System: The request call and response can traverse through multiple layers, preserving privacy by restricting the client and server from identifying the source of communication.
- Statelessness: Each client request is independent of another and does not influence the occurrence of one another. The data required to complete the requests is stored in the API call, eliminating the need for the server to store data related to client requests.
- Caching: The REST API supports the caching feature to enhance server response time. By storing frequently used responses, it eliminates redundant request processing.
- Code on Demand: One widely used principle of REST APIs is in designing web services. A server can send executable code to the client, which the client can run dynamically.
Benefits of Using REST API with Python
Let’s look at a few benefits of using Python REST API.
Scalability: REST APIs' statelessness optimizes client-server interactions by providing complete context to each request. If the server doesn’t require past information, REST API removes unnecessary workloads, reducing the server's overhead. When working with REST API in Python, client-server interactions are reduced by an efficient caching procedure, which enhances data retrieval time.
Speed: Leveraging REST API in a Python environment enables you to utilize asynchronous frameworks like asyncio to execute tasks simultaneously, enhancing performance. Another method to improve performance is using Python parallel processing libraries like multiprocessing to perform multiple tasks at once.
Platform Independence: REST API is independent of the technologies used on the server and the client side. For example, you can write client-side applications in Python without understanding the server-side processes, which might be written in another language. Changing the technology on either side doesn’t affect the communication between them.
Flexibility: Working with REST API provides you with more flexibility due to the decoupling principle between the client and server. Multiple layers between both the ends of the client and server hide the flow of data. You can even change the database layer without rewriting the application logic.
What Are HTTP Methods in REST API?

REST APIs use HTTP methods to determine what actions they are supposed to perform on the web server resources. These resources represent data available in a web service, which can be accessed and transformed according to the requirement.
To interact with REST APIs in Python, you can use a library, such as request, which empowers you to send HTTP requests to the server and receive responses. HTTP methods used in REST APIs like GET POST, PUT, PATCH, and DELETE encourage you to manage the state of resources available in the web server.
- The GET method enables you to retrieve data from an API. It supports read-only operations and does not allow data modification.
- The Post HTTP method lets you update or add information to the web service endpoint. Unlike GET, this option can be beneficial for altering existing resources rather than just performing read-only operations.
- Using the PUT method, you can replace existing resources with new data.
- You can use the PATCH HTTP method to modify certain values in an existing resource. As compared to PUT, this method doesn’t completely replace the existing data.
- If you wish to delete any resource, utilize the DELETE Python REST API method. This option is authenticated with an API key to restrict unauthorized access to your web server endpoints.
4 Use Cases for Using REST API with Python for Data Engineers
Here are a few of the prominent Python REST API use cases:
Data Pipeline Creation
Using REST APIs in Python is beneficial for automating extraction, transformation, and loading (ETL) operations between different sources. Building a data pipeline is crucial to generating impactful insights.
Example: A data pipeline migrates information from a finance management tool, like NetSuite, to a data warehouse like BigQuery. Here, Python can be utilized to extract data from the NetSuite API, apply business logic to it, and load it inside BigQuery in a single developer environment.
Real-Time Data Streaming
With Python REST API, you can fetch real-time data to deliver updated insights instantly. By leveraging Python’s streaming data libraries, like kafka-python and apache-flink, you can handle different data streams for applications.
Example: For banking applications, using real-time data streaming can be an essential step in identifying fraudulent transactions. Accessing data through a transactional database API in a Python environment and applying machine learning models, like logistic regression, can flag suspicious activities.
Database Management
Python REST APIs like DB-API 2.0 enable you to interact with database management systems to handle schema updates, monitor data, and create backups. Instead of writing separate code for managing different databases, this REST API lets you perform operations on multiple databases simultaneously.
Example: Centralize patient record management in a hospital database by creating a unified system that allows real-time data creation, updating, and sharing functionality. Use the GET HTTP method to retrieve schema information from the specified database, while POST to activate backup triggers.
Exploratory Data Analysis
By extracting data using REST API in Python, you can perform advanced analytics on it to produce actionable business insights. Creating strategies from these insights can enable you to enhance performance and customer satisfaction.
Example: Extracting data from e-commerce tools, like Shopify, to analyze past customer transactions. The results will encourage the creation of effective marketing campaigns catering to customer’s specific needs.
For another example, consider extracting raw coin data from a cryptocurrency exchange to visualize a portfolio, representing net profit from each coin.
Python Libraries for Building REST APIs
The key reason why Python is used to develop REST APIs can be attributed to the wide range of libraries and frameworks it offers. Let’s explore some of the most widely used REST API Python libraries.
Flask RESTful

Flask RESTful provides customizable, lightweight functionality, allowing you to build APIs that are flexible and fast. This micro-framework is easier to use if your existing web applications are built using Flask. Initially developed by Twilio, Flask RESTful can facilitate powering your public and internal APIs.
Django REST
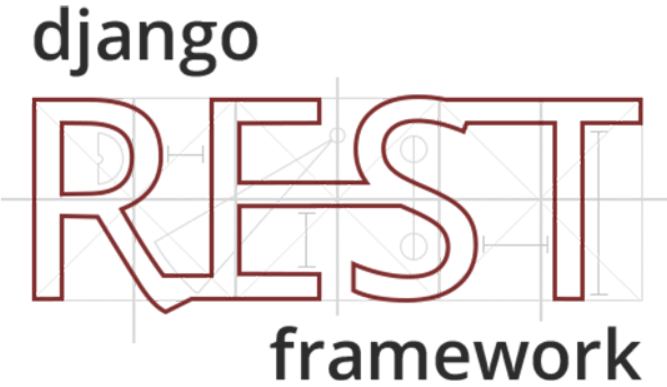
Django REST framework is a Python REST API library that can be used to effectively work with Django models to build powerful APIs. It contains pre-built features, such as ViewSet and Serailizers, that can save time and effort when creating APIs. With its serialization engine, you can transform complex Python objects into commonly used data types like JSON and XML.
FastAPI

FastAPI is a modern, high-performance web framework that is designed to be easy to use for building Python REST APIs. It supports asynchronous code, allowing multiple tasks to process simultaneously without compromising on performance. With support for Python-type hints, FastAPI aids in automatically validating incoming data by defining request and response data structure.
Falcon
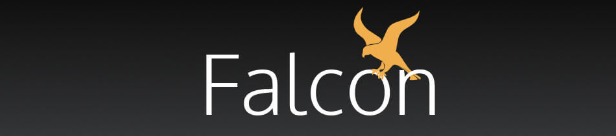
A minimalist ASGI/WSGI framework like Falcon lets you build mission-critical APIs and microservices. It offers a clean design, embracing the REST architectural model while focussing on reliability, accuracy, and performance at scale. Including built-in support for HTTP caching, Falcon allows you to optimize performance by reducing server load.
Pyramid

Pyramid is a full-stack web framework that offers multiple architectural patterns, including Model-view-controller (MVC) and Model-view-presenter. With this framework, you can build APIs based on your specific requirements. Features like routing, views, authentication, and authorization provide you with flexibility and versatility when developing REST APIs.
Utilizing Pyramid’s routing functionality, you can define URL patterns and map them to views that generate responses. This helps dynamic behavior support based on request parameters and matched routes.
Bottle

Bottle is a Python REST API framework usually used for small and medium-sized web applications. Its support for developing applications with embedded web servers makes it easy to deploy without requiring a separate web server. Distributed as a single-file module, Bottle has no dependencies other than the Python Standard Library. Prototyping simple ideas in this framework is comparatively easier than in opinionated web libraries like Django.
Using REST API with Airbyte
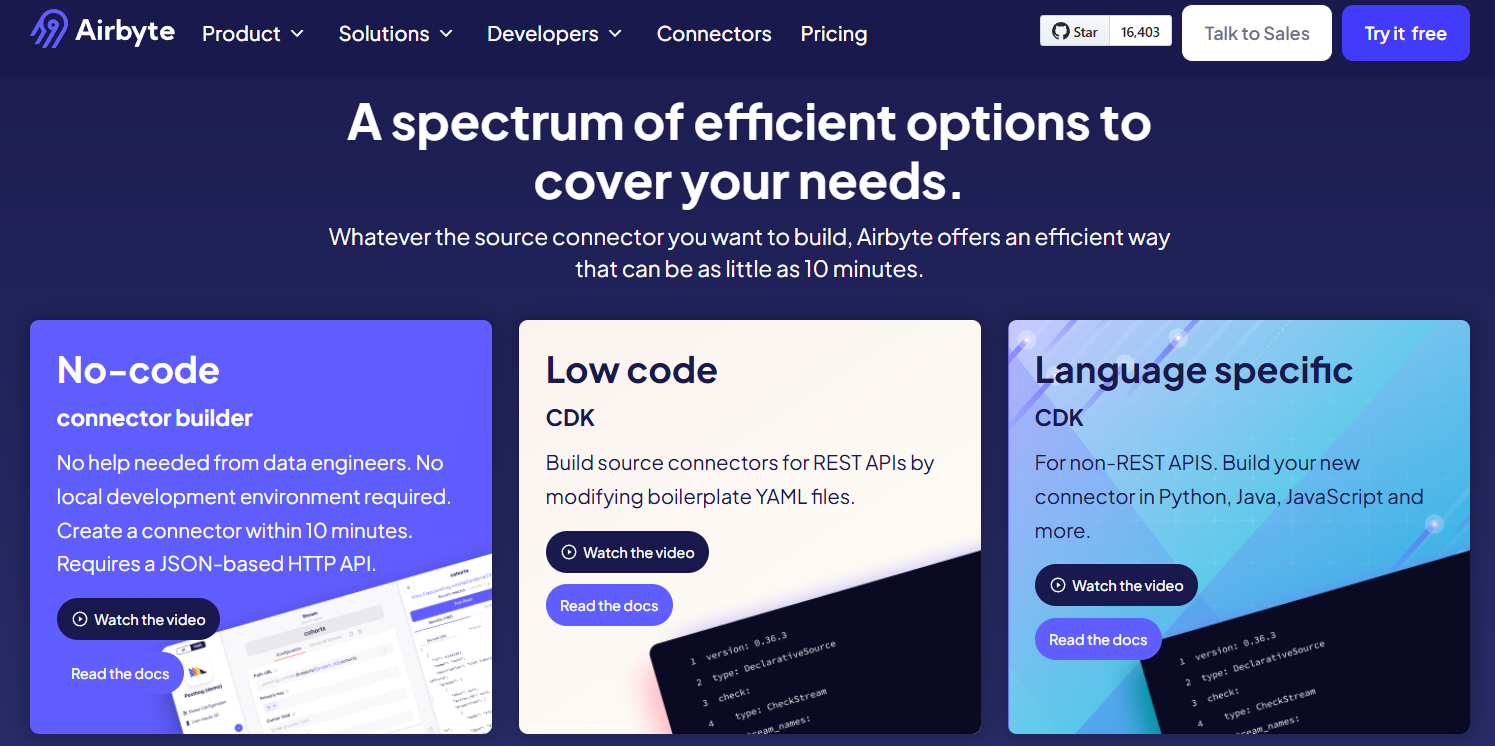
In real-world applications, data is often spread across different platforms, from marketing tools to resource planning systems. Consolidating this data into a centralized repository is advantageous for streamlining data-driven decision-making. However, manually performing data migration can be challenging and require certain technical expertise. To overcome this complexity, you can leverage tools like Airbyte for effective data transfer.
Airbyte is a robust data integration tool that enables you to move data from various sources to a destination of your choice. With over 550 pre-built connectors, it allows you to replicate structured, semi-structured, and unstructured data between different platforms. If the connector you seek is unavailable, you can use Airbyte Connector Development Kits (CDKs) or Connector Builder to develop custom connectors.
Here are a few valuable features offered by Airbyte:
- Simplified REST API Interactions: Airbyte’s Public APIs source connector gives access to the most publicly available APIs that you can use to fetch data from numerous external sources. It supports full refresh syncs, allowing retrieval of all available data, regardless of previous data synchronization operations. For example, you can migrate data from Public APIs to Amazon S3 for enhanced scalability and analytics capabilities.
- AI-powered Connector Builder: Airbyte Connector Builder comes with an AI assistant that reads through your preferred platform’s API documentation to auto-fill most configuration fields. This significantly simplifies your connector development journey.
- Change Data Capture (CDC): CDC enables you to identify incremental data changes made to the source platform and replicate them in the destination systems. This allows you to keep track of updates and maintain data consistency.
- Support for Vector Databases: Airbyte supports prominent vector databases, inducing Pinecone, Weaviate, and Chroma. You can use RAG techniques, such as chunking, embedding, and indexing, to convert your data into vector embeddings. Storing these embeddings in the supported vector databases streamlines the development of AI applications.
- Self-Managed Enterprise Edition: Using Airbyte Enterprise Edition, you can handle large-scale data workloads in your own Virtual Private Cloud (VPC). It offers features like multitenancy, role-based access control, personally identifiable information (PII) masking, and enterprise support with SLAs, providing you with enhanced control and security.
- PyAirbyte: Airbyte offers a Python library, PyAirbyte, which enables you to leverage the Airbyte connectors to extract data from multiple sources into prominent SQL caches. These caches are compatible with numerous Python libraries, such as Pandas, and AI frameworks, like LangChain.
You can also use Airbyte to build custom connectors for REST APIs. This provides an alternative to building data pipelines in the development environment, which can often be complex. By providing the necessary connector details in the Airbyte Connector Builder, you can create custom connections within a matter of minutes. These connectors simplify data replication between different tools used within your organization, enabling you to centralize data for better accessibility.
Conclusion
Using Python REST API is an effective way to enable seamless communication between different applications within your workflow. REST API’s stateless nature offers independence between different API calls, while Python provides robust features to handle raw data.