Text Analysis in Python: Techniques and Libraries Explained
Analyzing text data, whether it is online reviews of your products or feedback from your clients, is crucial. Performing this analysis helps you create better business solutions that cater to your customer’s specific requirements. However, conducting text analysis can often be daunting, requiring technical expertise and domain knowledge.
In this article, you will explore the concept of performing text analysis in Python, with different methods that you can use to streamline insights generation.
Getting Started with Text Analysis in Python
The biggest challenge analysts face when analyzing text data is data quality. Most real-world applications produce messy and noisy data. Cleaning and transforming this data into an analysis-ready format is an essential step. This is the key reason why you must use Python for text analytics.
Python offers different data structures that allow you to work with data in any format. Its support for robust machine learning and natural language processing libraries enhances your analysis experience, enabling you to perform advanced analytics.
The first step in Python text analysis is understanding the data you want to analyze. A comprehensive overview of the text data characteristics, including its format and structure, is essential for analysis. By recognizing the patterns and formats within the text data, you can proceed with the data extraction steps. This knowledge allows you to scrape data from a website or extract it from another tool into the Python environment effectively.
After getting a brief overview of the data you will be working with, it is time to set up Python on your local machine and create a virtual environment. You can download and install Python from the official website.
To create and activate a Python virtual environment in Windows, execute the below command in the command prompt:
Performing the same operation in the Mac command line interface (CLI) goes like this:
Now that you have created a virtual environment, you can extract the data into your local machine. The data you will be working with might be available in dispersed sources. If your task requires scraping data from a webpage, you can use Python libraries like Requests and BeautifulSoup. In hindsight, if your workflow requires extracting data from dispersed sources into a centralized location for text analysis, SaaS-based tools like Airbyte can be beneficial.

Airbyte is a no-code data integration tool that allows you to migrate data from multiple sources to your preferred destination. With more than 400 pre-built connector options, it offers you the flexibility to perform text analysis on data extracted from numerous platforms.
If the connector you seek is unavailable in the available options, Airbyte provides you with a no-code Connector Builder and Connector Development Kit (CDK). The Connector Builder has an AI assistant that reads through your preferred platform’s API documentation and fills out most fields in the user interface. This simplifies your connector development process, allowing you to build custom connectors within minutes.
Here are a few features offered by Airbyte:
- Streamline GenAI Workflows: Airbyte enables you to extract raw, unstructured, and semi-structured data and convert it into vector embeddings using RAG transformations. These vector embeddings can be stored in Airbyte-supported popular vector databases like Pinecone, Milvus, or Qdrant to simplify genAI workflows.
- PyAirbyte: Airbyte offers a Python library, PyAirbyte, which you can use to directly load data from different platforms into SQL caches using Airbyte connectors. You can convert these SQL caches into Pandas DataFrame to work with the data in a Python environment. By using Python’s capabilities to transform data, you can perform sentiment analysis on any dataset.
- Large-Scale Workload Management: With its Enterprise edition, Airbyte allows you to manage large-scale data workloads. Features like multitenancy, role-based access control (RBAC), enterprise support with SLAs, and personally identifiable information safeguard your data from unauthorized access.
Essential Libraries Overview
Python has extensive libraries that enable you to perform natural language processing tasks. With the help of these libraries, you can produce insights from textual data. Let’s explore the most essential libraries for conducting text analysis in Python.
NLTK
Natural language toolkit, or NLTK, is an open-source Python library that is most widely used for text analysis. It offers you easy-to-perform functions, including stemming, tokenization, lemmatization, parsing, and sentiment analysis. The advantage of using NLTK is that it provides a diverse set of corpora, including various sources like books, articles, and social media posts. These corpora simplify the training and testing of NLP models.
spaCy
spaCy is an industry-scale NLP library offered by Python that is written in memory-managed Cython, a combination of C and Python. By offering a variety of plugins, spaCy allows you to integrate data with your machine learning stack to build custom workflows.
TextBlob
TextBlob is a text processing Python library that provides a simple API to perform common NLP tasks, such as part-of-speech tagging, sentiment analysis, and classification. It offers features like n-grams, word inflection and lemmatization, spelling correction, and WordNet integration to improve your language processing steps further.
Scikit-learn
Scikit-learn is a free, open-source Python library that supports the most popular machine learning algorithms, including linear regression, logistic regression, and random forest. It offers a wide range of features that can help you transform data and build your own machine learning model trained on it.
Text Preprocessing Fundamentals
After selecting the libraries that best fit your specific requirements, the next step is to understand the fundamentals of text preprocessing. In this section, you will explore the preprocessing techniques to follow for enhanced text analysis.
Tokenization Techniques
Tokenization is the process of breaking down large, complex text into individual words or subwords, also known as tokens. Breaking down large chunks of text into smaller components allows text processing libraries to understand the structure, meaning, and context of the language. For example, if you perform word tokenization on this sentence: “Humpty Dumpty sat on a wall,” the results will be an array of words.
Output:
['Humpty', 'Dumpty', 'sat', 'on', 'a', 'wall']
Tokenization is broadly classified into three types: word, character, and subword tokenization. Word tokenization splits the text into different words. The character tokenization breaks the text into individual characters. Subword tokenization, on the other hand, splits texts into components that are larger than a single character but smaller than a word.
Stop Word Removal
When performing text analysis in Python, it is necessary to remove stop words from the tokenized text. Stop words do not add any significant value to the contextual meaning of the text. Eliminating the unnecessary words allows you to focus on relevant content and extract meaningful insights from the data.
For example, let’s consider another sentence: “Text analysis is as easy as climbing a wall for Humpty Dumpty.” You can execute the code mentioned below to remove stop words from this sentence.
Output:
['Text', 'analysis', 'easy', 'climbing', 'wall', 'Humpty', 'Dumpty', '.']
Stemming vs. Lemmatization
Stemming and lemmatization are popular Python text analysis techniques that convert raw text data into a structured format.
Stemming is the process of removing suffixes from a word to get its base form, which might not be an existing dictionary word in a language. It relies on the current word when processing without any knowledge of the context of the text. For example, the word “removing” can be converted into “remov.” To perform stemming using NLTK, you can use PorterStemmer.
Contrarily, lemmatization is the process of reducing the characters from a word to get its root form, or lemma, which is a proper dictionary word. It uses the context of other words to output the correct lemma from a word. For example, the word “removing” is transformed to “remove.” To perform lemmatization in NLTK, you can utilize WordNetLemmatizer.
Handling Special Characters
During text data preprocessing, it is important to remove punctuations and special characters that do not add value to the resulting insights. Retaining the alphanumeric characters and spaces between the words reduces the processing time and complexity.
RegEx, or regular expression, is a prominently used library that helps you eliminate special characters from a text. Working with RegEx requires you to import the Python “re” library.
Case Normalization
Case normalization is the step of converting all the characters in a text in either uppercase or lowercase to promote standardization of each letter. To convert the text in a string into lowercase or uppercase, use the .lower() and the .upper() Python built-in methods, respectively.
Dealing with Multilingual Text
Before starting with text analysis in Python, you must handle the presence of multiple languages in text. If multilingual text is not handled properly, the results of the model might be totally biased or even incorrect. You can identify the language of each text using libraries like langdetect, fastText, or NLTK algorithms like TextCat.
Basic Text Analytics
Preprocessing the text data prepares it for further analysis. The next step is to perform basic analytics on the text data, such as word frequency analysis, readability scores, and basic statistics. This initial analysis step will provide you with an overview of the trends and patterns within the data.
- Word Frequency Analysis: Understanding the number of times a word appears is essential in Python text analysis. To get the word frequency from any text, you can build a custom function that loops through all the words in the tokenized list. Creating an empty hash function or dictionary and storing each word with its count as a key-value pair can help you perform word frequency analysis.
- Vocabulary Richness: Vocabulary represents the set of unique words that appear in the text corpus, signifying text richness in terms of the variety of new words. It also outlines the number of words that you must transform into machine-compatible vectors. One common way to get the vocabulary of the data is by converting the tokenized list into a set.
- Readability Scores: In NLP, readability scores highlight the ease with which the users can understand the content. Readability relies on the vocabulary and the syntax of the text involved. There are multiple ways to determine the readability score of any text, including Flesch Scores, the Gunning Fog Index, the SMOG Readability formula, and more.
- Basic Statistics: In this step, you can also perform statistics to understand the nature of the data you are working with. For example, you can calculate the mean length of documents in a dataset to detect anomalies like short-length or lengthy documents.
- Pattern Matching with ReGex: ReGex allows you to specify the pattern of text to look for in the corpus. It can detect text availability by comparing the mentioned pattern with the original data. Using ReGex, you can also split the pattern into sub-patterns to get a better understanding of the data.
- N-Gram Analysis: An N-gram outlines the basic context of the data, mentioning what word or letter will follow the available information. The value of N determines the amount of information available. For example, N = 1 is a unigram, N = 2 is a bigram, and N = 3 is a trigram. The higher the value of N, the better the analysis model will capture language structure.
Sentiment Analysis
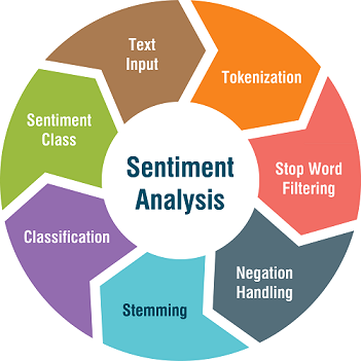
Sentiment analysis is a crucial step of text analysis workflow as it captures the user behavior. A primary real-world example involves performing sentiment analysis on product reviews that provide an overview of the user experience.
Many businesses, including online shops registered on E-commerce websites like Amazon, Airlines such as Emirates, and YouTube creators, employ analytics teams to analyze user reviews. This helps enhance the user experience and improve goods and services to cater to users' needs.
Here are a few methods and terms to perform sentiment analysis on your data:
Rule-Based Approaches
Also referred to as lexicon-based analysis, this approach involves defining a set of predefined rules to determine the sentiments of text data. It is called so because the rules followed are based on synthetic or lexical features of the text. While it is an easy-to-perform approach, it might not produce effective insights when working with more complex data.
Machine Learning Methods
This method involves identifying the sentiments of a piece of text using models that are trained on labeled data. The models can comprise any machine learning algorithm, including decision trees, support vector machines (SVMs), neural networks, or an ensemble of all these. Compared to the rule-based approach, this method is better suited for large datasets. However, it can often be computationally expensive to perform.
Polarity Detection
It is a key aspect of sentiment analysis that highlights users’ feelings and opinions about new products or services. Generally used for social media sentiment analysis, polarity detection outlines a user’s positive, negative, or neutral reaction towards something.
Subjectivity Analysis
Subjectivity in sentiment analysis represents the extent to which the text expresses personal attitudes, opinions, and feelings. It is a more nuanced way of highlighting sentiments. The objectivity of a sentence highlights the factual correctness, while the subjectivity discusses personal experiences.
Emotion Detection
It is the process of determining various human emotion types, such as anger, fear, joy, and excitement. By analyzing the emotions of your users, you can enhance business processes to fulfill their specific requirements.
Handling Negations
Negative words like not, never, no, shouldn’t, cannot, and many more can affect the sentiments involved in a text. Negation handling is the process of identifying the extent of negative emotions and inverting the polarity of the text under the influence of the negation. To handle negations, you can use Python’s NegSpacy library.
Let’s explore a real-world use case of sentiment analysis.
In this example, we will leverage Airbyte to extract data from Intercom—a customer service platform—into Google BigQuery—an analytics platform. The next step uses MindsDB, an AI tool that integrates with BigQuery and allows you to perform sentiment analysis on the data. Finally, the analysis can be visualized using BI tools like Metabase.
The first step involves migrating data from Intercom to BigQuery. To achieve this, you can login to your Airbyte account and configure the source and destination.
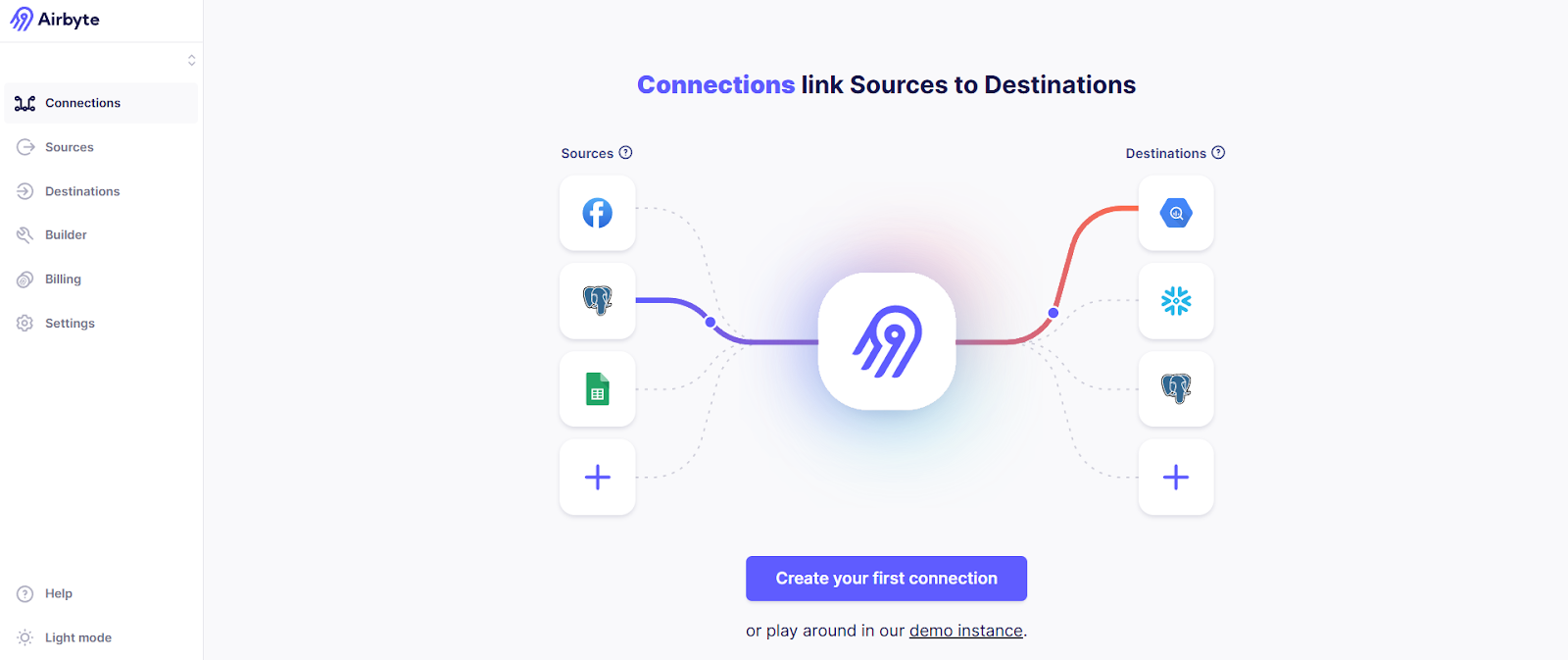
By clicking on the Connections tab and mentioning all the necessary fields, you can set up this integration. Within a few minutes, you will be able to view the Intercom data in your mentioned BigQuery location.

Now that the data is available in BigQuery, the next step is to enrich it with user sentiments. For this, you can utilize MindsDB and its OpenAI integration to power your database with the capabilities of GPT-4. MindsDB’s support for SQL makes it a robust, easy-to-use platform.
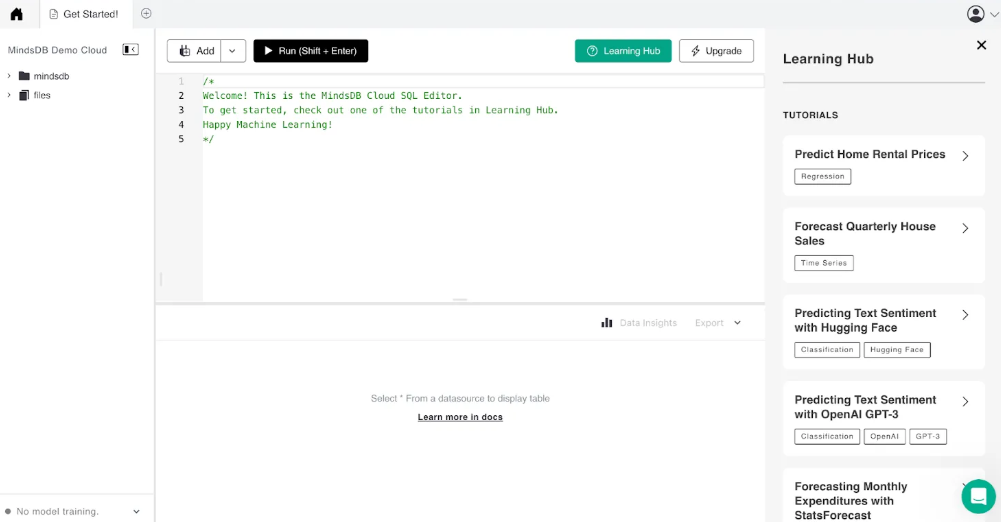
After querying the data and analyzing the user sentiments, migrating the insights back to BigQuery is a beneficial step for producing visualizations. The final step requires you to build a dashboard with the help of Metabase that represents the insights in a highly intuitive form.

Following these easy steps can enable you to visualize the sentiment analysis of customer data. To learn more about the steps involved, refer to the official tutorial.
Named Entity Recognition (NER)
NER is the process of recognizing and classifying named entities, including names, locations, dates, and times from raw data. Performing NER enables you to retrieve information from huge text datasets. Some commonly used named entities are organization names like NVIDIA and APPLE, locations like Delhi and Mumbai, and dates like 15th November 2024. Here are the most commonly used NER types:
- Person Identification: In this scenario, the NER identifies a person’s first, middle, and last names, honorifics, and titles. For example, Dr. Jane Doe.
- Organization Detection: This form of NER detects the institution’s name from a piece of text, such as Meta, the United Nations, or FIFA.
- Location Extraction: It involves recognizing any location’s name, including countries, states, cities, and landmarks, such as New York, London, and Bengaluru.
- Date/Time Recognition: This method extracts date formats and identifies time expressions, such as 2:10 PM, 17:00, and 2024-01-05.
- Custom Entity Training: The custom entity training allows you to identify your domain-specific entities from unstructured data. Customizing the NER enables precision in extracting relevant information.
- Entity Linking: In this process, the named entities are recognized and associated with a knowledge base for better accessibility and contextual meaning.
Text Classification
Text classification is the process of classifying open-ended text into a number of predetermined categories. It enables you to structure, categorize, and arrange raw text data from different sources, including articles, news pieces, stories, etc. Classifying this data into multiple classes allows you to streamline the extraction of meaningful insights. Let’s explore the various ways to classify text.
Feature Extraction Methods
In NLP, this step revolves around transforming raw text data into a machine learning-compatible format. There are numerous techniques available to perform feature extraction in NLP. Some of the common techniques include:
TF-IDF Implementation
Time Frequency-Inverse Document Frequency is a prominent statistical measure that assigns weights to words in a text corpus based on their importance. It assigns a numeric value to words by calculating the product of term frequency and inverse document frequency.
The term frequency represents the frequency of a word in a document. In contrast, the inverse document frequency signifies the logarithm of total documents divided by the number of documents in which the word is repeated. Thus, The TF-IDF vector represents each document as a vector in a multidimensional space.
Word Embeddings
In this feature extraction method, each word is represented as vectors in a high-dimensional space. Similar words are closer to each other, while dissimilar words are located far apart.
Word embedding models usually utilize unsupervised learning algorithms like GloVe or Word2Vec and are trained on huge amounts of data. These models use neural networks and determine the weights and biases that best work with original data. After training, the model can be used for multiple tasks, such as sentiment analysis and text classification.
Document Classification
The underlying technique involved in classifying documents is Latent Dirichlet Allocation (LDA). LDA assumes that each document in the corpus is a combination of topics, where each topic is a probability distribution over words. It assigns each document’s words to topics and accordingly adjusts the topic-word probabilities, resulting in a list of topics containing words defining them.
Multi-Label Classification
Multi-label and multi-class text classification might sound similar to each other, but they are significantly different. In a multi-class classification, a token can only belong to a single class from a set of multiple classes. In contrast, multi-label classification allows you to assign multiple classes to a single token.
An example you might consider is that a movie can be classified as horror and thrilling at the same time. This example assigns a text piece with different labels that help enhance the understanding of the information.
Model Evaluation Metrics
After performing text analysis in Python by classifying the objects in a text, the next consideration is the reliability and accuracy of the model. The accuracy of any machine learning model defines how well the model predicts the outcome of the data. The key text analysis Python evaluation techniques involve recall, accuracy, precision, and f1-score.
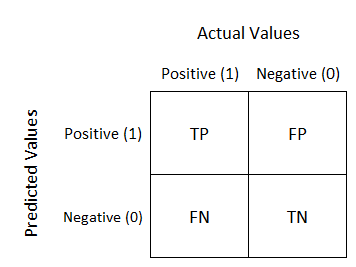
Recall highlights the ratio of correctly classified actual positive values to all actual positive values. Accuracy defines the ratio of correct classifications to the total classifications. Precision is the ratio of correctly classified actual positives to the total observations classified as positive. F1-score is the harmonic mean of precision and recall.
Advanced Text Processing
Let’s explore some of the advanced text processing techniques that can be useful in certain scenarios.
Text Summarization
The process of summarizing text content in simpler, more compact words is known as text summarization. Libraries like spaCy and PyTextRank can be combined to provide you with the capability to create a text summarizer. To further enhance the summarizer, you can develop an abstractive summarization technique using HuggingFace’s Transformer library.
Keyword Extraction
The method of extracting the most relevant keywords from a piece of text is called keyword extraction. By extracting the keywords from text data, you can retrieve essential information associated with the given text. Libraries like RAKE, YAKE, KeyBert, and NLTK’s TextRank-based keyword extraction enable you to access keywords.
Cross-Lingual Analysis
It is the specialized part of text analysis that uses resources from different languages, especially when the data in a certain language is limited. Cross-lingual analysis uses methods like transfer learning to enable models to learn languages with extensive amounts of data, like English. The attained knowledge is then transferred to languages with less data availability. Performing this analysis utilizes bilingual lexicons, word embeddings, and pre-trained models.
Text Generation
Training neural networks, statistical, and transformer-based models allow you to create a text generator. By learning the patterns in the training data, the model becomes efficient in producing original content. To generate new content, statistical models involve n-gram models and conditional random fields (CRFs). Neural networks use recurrent neural networks (RNN) and long short-term memory (LSTM) networks. And transformers use generative pre-trained transformers (GPT) or bidirectional encoder representations of transformers (BERT).
Working with Large Text Datasets
By now, you must have understood the methods of performing text analysis in Python. In real-world applications, you might be working with large datasets that consume a lot of processing time and resources. To overcome challenges like these, you can follow a few best practices that improve analytics workload management and performance.
- Efficient Text Processing: Before starting with the Python text analysis steps, you must thoroughly conduct data processing steps. Efficiently processing data ensures the removal of nonessential components that might hinder the model performance when working with large datasets.
- Parallel Processing: One of the best ways to optimize analytics performance is distributing the workload across multiple nodes. This improves the parallel processing of tasks and reduces the time consumed in performing tasks sequentially.
- Memory Optimization: To get the best performance, you must optimize memory allocation. Techniques such as columnar storage, data partitioning, and data compression can play an important role in enhancing the processing speed.
- Batch Processing: Processing large datasets in batches is a crucial step in optimizing the model performance. For large amounts of data, performing analysis in batches is often better than stream processing, allowing better resource management while reducing additional overhead.
- Scaling Strategies: Another beneficial step for handling large text datasets is creating scaling strategies to manage data effectively. Concepts like sharding, partitioning, distributed computing with Spark and MapReduce, and dimensionality reduction play a crucial role.
- Performance Monitoring: After conducting all the performance optimization steps, it is necessary to monitor the data processing task continuously. This may involve tracking memory usage, CPU utilization, key metrics, etc.
Text Visualization
The final step of any analysis, including text analysis, is the visualization of the insights generated. This can involve different ways to express the message conveyed by the outcomes.
Visualizations effectively highlight complex findings in the analysis step in a simple-to-understand way. By visualizing the results of the analysis, you can represent technical concepts on a dashboard that the stakeholders can easily navigate.
Here are the ways in which you can visualize the Python text analysis results:
Word Clouds
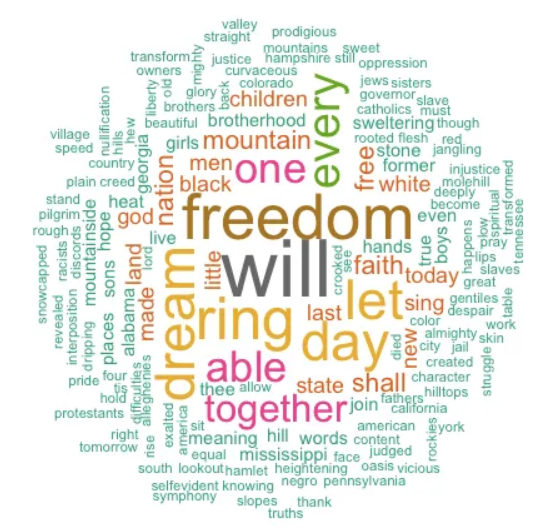
A word cloud is an effective way to represent the appearance and importance of words in the analyzed text. The importance of any word is demonstrated by the number of times it appears in the document.
Network Graphs
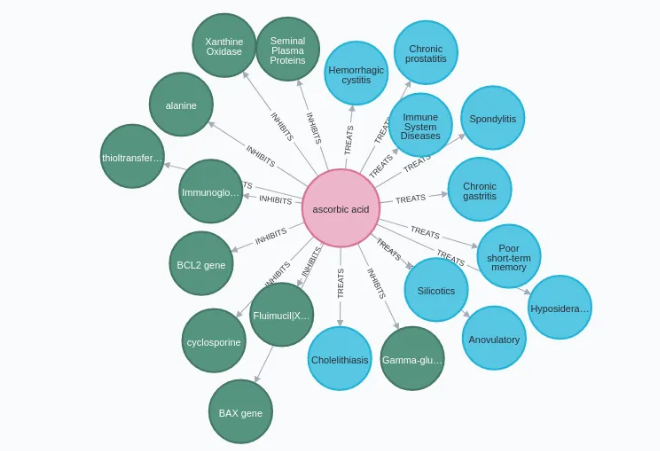
Network graphs are visual representations of different words or concepts in any document and the links between them. They are a useful way of representing data and its relationships.
Topic Visualization
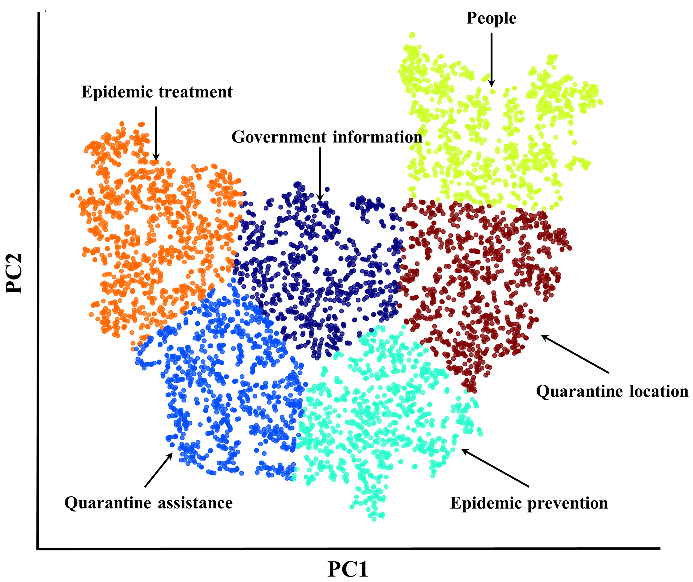
Topic visualization is the process of creating structured data from unstructured raw data. You can consider topic visualization as the representation of random data points into different classes using unsupervised learning.
Trend Analysis Plots
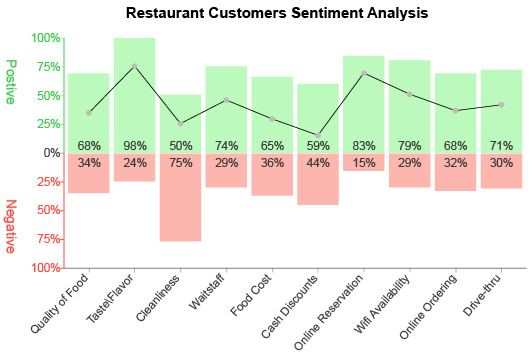
Trend analysis plots are used to represent the trends involved in the text data. With this visualization method, you can highlight the sections of your business where you are doing well and where improvements are needed.
Interactive Dashboards
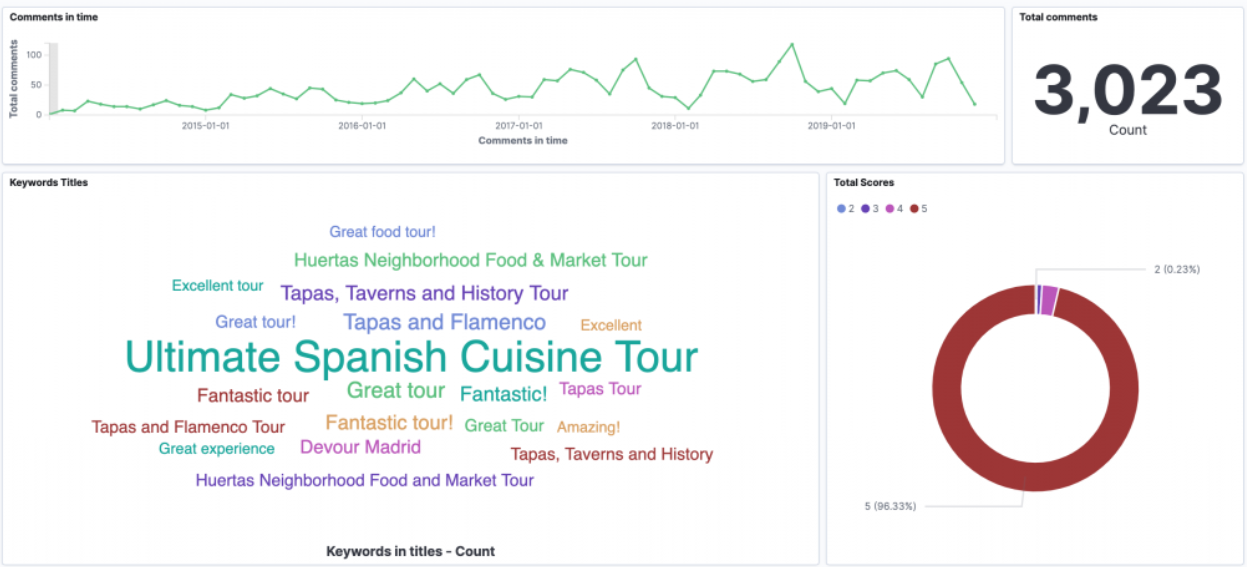
Building interactive dashboards is a great way of representing the data that your users can interact with. This enhances the experience of working with data while outlining the key terms businesses must focus on.
Custom Visualizations

Another great way to present data in a simple manner is to use the capabilities of Python’s Matplotlib or Seaborn libraries. While these tools require extensive programming knowledge, you get the flexibility to represent the data according to your preference.
How Is HuggingFace Paving the Way for Text Analysis with Its Open Source Offering?
HuggingFace is an open-source tool that works as an ecosystem, offering robust capabilities for performing machine learning and deep learning tasks. Founded in 2016, the company’s main focus was on developing chatbots. However, HuggingFace switched its objective to a wider niche of machine learning, providing developers the opportunity to work with the latest AI models.
Offering a wide range of free-to-use features, HuggingFace has enhanced the accessibility of text analysis for a global population. Features like easy access to community models and datasets, as well as the ability to implement and fine-tune machine learning models, enhance user experience. HuggingFace’s support for research and evaluation further helps users when working on complex problems.
Over the recent years, HuggingFace has revolutionized how researchers, developers, and even students used to work with text data for analysis.
Key Takeaways
Text analysis in Python is a great way to generate insights and visualize data to create effective marketing strategies. With the increasing number of methods and tools to perform text analysis, choosing the correct one becomes essential. To select the best analysis method, you must thoroughly understand the data you will be working with and the best practices to optimize performance.