What Are Data Structures & Their Types
Data structures are the backbone of computer science, providing the foundation for organizing, storing, and retrieving data efficiently. Understanding the types of data structures and their characteristics is essential for software developers, data engineers, and anyone working with complex data systems. This guide will take you through the different types of data structures, key operations, time and space complexity, and how to choose the best data structure for your project.
What Are Data Structures?
A data structure is a way of organizing and storing data in memory so that it can be accessed and modified efficiently. It provides a systematic way of managing data that allows for quick and efficient operations such as searching, inserting, updating, or deleting data.
In computer programming, choosing the right data structure can drastically improve the performance of your system. Whether you're working with operating systems, databases, or mobile apps, understanding how to leverage data structures will help you build faster and more scalable systems.

Data structures can be classified into various types of data structures based on their structure, behavior, and operations. These include linear data structures like arrays and linked lists, non-linear data structures such as trees and graphs, and more specialized hash data structures like hash tables and bloom filters.
Why Are Data Structures Important?
Data structures are crucial because they allow for efficient problem-solving in a variety of ways:
- Handle Different Data Types: Data structures let you handle multiple data types (text, numbers, images) within the same abstract data types.
- Boost Developer Productivity: Many programming languages provide libraries for common data structures, reducing the need to reinvent the wheel.
- Improve Runtime: The right data structure can significantly improve the speed of retrieving data, making systems more responsive.
- Scalability: Data structures that are well-suited to handle large volumes of data points help maintain performance even as traffic or data grows.
- Clean Abstraction: They enable clear separation between business logic and data storage, making code easier to maintain and understand.
Operations on Data Structures
Data structures support a range of operations to manipulate data:
- Search: Finding an element that meets a specific condition.
- Insertion: Adding new data elements.
- Deletion: Removing data items from the structure.
- Traversal: Visiting each data element in the structure in a systematic way (in order for linear data structures or hierarchically for trees).
- Sorting: Organizing data values for quicker future retrieval.
- Update: Modifying existing values.
Data Structure Classification
Understanding the classification of data structures is essential for selecting the right one for your project. Data structures can be broadly categorized based on their organization, memory usage, and how elements are arranged. Each category serves specific needs and offers unique advantages depending on the type of data you’re working with and the operations you need to perform. Let’s dive into each category and look at the most common data structures within it.
1. Linear Data Structures
Linear data structures store data in a sequential arrangement and are useful for scenarios where order matters.
Array Data Structure
An array data structure is a collection of data items of the same type stored in contiguous memory locations. It allows fast O(1) random access, making it ideal for numerical computing and image buffers.
Stack Data Structure
Stacks follow the Last-In, First-Out (LIFO) principle. They are used for function call management and parsing expressions, with operations such as push, pop, and peek.

Here are some other basic stack operations :
- Peek: View the top element without removing it from the stack.
- IsEmpty: Verifying whether the stack is empty.
- IsFull: Verifying whether the stack has reached the maximum capacity.
Stacks are highly advantageous in data management scenarios where the sequence of operations is more significant.
Queue Data Structure
Queues implement the First-In, First-Out (FIFO) principle. They are typically used for tasks such as print spooling, message brokering, and pipeline buffering.

The queue processes the data in the order they were received. An example of queues is job scheduling in operating systems. Queues are used to schedule and prioritize jobs waiting to be executed by the system. Then, jobs are processed in the order specified at the time of arrival.
Here are some basic operations performed on a queue:
- Enqueue: Used to insert an element at the rear end of the queue.
- Dequeue: Used to delete an element from the front end of the queue.
- Peek: Returns the element, which is pointed by the front pointer in the queue.
- Queue Overflow: The overflow condition is shown when the queue is full.
- Queue Underflow: The underflow condition is shown when the queue is empty.
Linked List Data Structure
A linked list data structure consists of nodes where each node contains data and a pointer to the next node. This dynamic data structure is perfect for frequent insertions or deletions without shifting other elements.

Linked lists can grow dynamically, making them suitable in scenarios where the data size is unknown.
2. Non-Linear Data Structures
Non-linear data structures are used to represent hierarchical relationships or complex networks.
Tree Data Structure
A tree data structure represents hierarchies with a root node, parent nodes, and child nodes. It is often used in file systems and databases.

In the above tree structure, each node is labeled with a name.
- Root Node: It is the topmost node in the tree data structure with no parent. In the above example, node A is the root.
- Parent Node: The parent node is any node with child nodes directly under it.
- Child Node: If the node descends from a parent node, it is termed a child node.
- Siblings: The sibling nodes are those with the same parent.
- Leaf or External Node: Leaf nodes have no child nodes. In the above example, F is a leaf node.
- Internal Node: A node with at least one child node is termed an internal node.
- Ancestor Nodes: A node's ancestors refer to any nodes preceding it on the path to the root. For example, nodes A and B are ancestors of node E.
- Descendants: These are nodes accessible by traversing downwards from a given node in a tree. In the above data structure, nodes D and I are the descendants of node B.
- Subtree: It refers to a portion of a tree that includes a node, also referred to as the root of the subtree, and all of its descendants. For example, if node B is the root of a subtree, then nodes D, H, and I comprise the descendants of the subtree.
Graph Data Structure
A graph data structure consists of vertices (nodes) connected by edges, and it is used to model networks, such as social graphs or dependency trees.
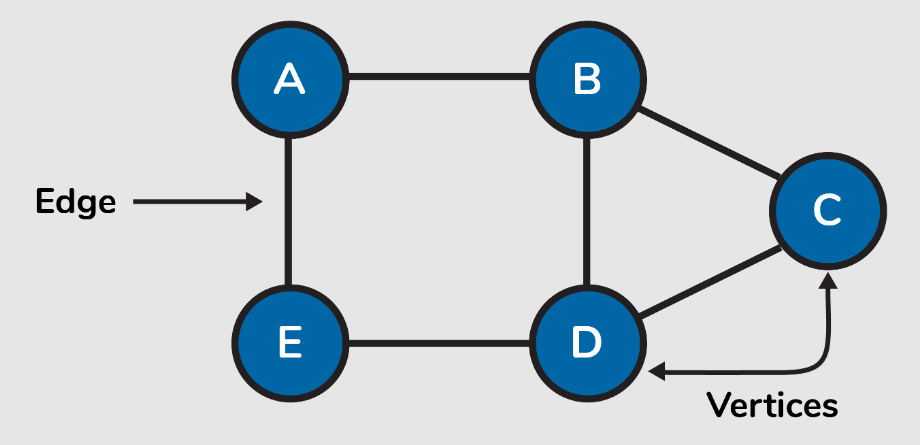
Graph components include:
- Vertices: Vertices, also called nodes, are a graph's basic components. Each vertex or node may be assigned a label or remain unlabeled.
- Edges: Edges serve as connections between two vertices in the graph. Edges connect any two vertices in a graph, and similar to vertices, edges may be labeled or remain unlabeled. In a directed graph, they can be represented as ordered pairs of vertices, whereas in undirected graphs, edges indicate a bidirectional relationship.
Binary Search Tree
A specialized binary tree where left < parent < right. It allows fast, ordered retrievals and is useful for range queries.
Applications of Data Structures
Data structures are a fundamental tool in computer science, designed to store, retrieve, and manipulate data in software applications. They can solve problems and be applied in various fields. Let us look at some of the practical applications of data structures:
- Dynamic Programming: Data structures like arrays are extensively used in dynamic programming to store the results and optimize the algorithms. For example, the matrix chain multiplication algorithm relies on arrays to efficiently store and retrieve data.
- Job Scheduling: Data structures like queues are used in task management systems for job scheduling. The first-in-first-out (FIFO) functionality of queues enables the orderly execution of jobs.
- Bioinformatics: Data structures like graphs are used to model and analyze complex biological networks, such as gene regulatory networks and protein-protein interaction networks.
3. Hash-Based Data Structures
Hash-based structures are designed for fast data retrieval through hashing.
Hash Tables/Hash Maps
Hash tables use a hash function to map keys to array indices. They provide near O(1) average time complexity for search, insertion, and deletion.
Sets & Hash Data Structures
A set stores unique elements, often implemented on top of hash tables, and is used for quick membership checks and duplicate elimination.
Bloom Filters
Bloom filters are probabilistic data structures that test membership with ultra-low memory overhead. They are commonly used in distributed systems to avoid unnecessary disk reads.
4. Specialized & Other Data Structures
Specialized data structures are optimized for specific use cases.
Heaps & Priority Queues
A heap is a tree-based structure that maintains a heap property (max-heap or min-heap), which is essential for implementing priority queues used in job scheduling and resource management.
Tries (Prefix Trees)
Tries are efficient for storing strings by common prefixes and are commonly used in autocomplete systems, IP routing, and dictionary lookups.
Time & Space Complexity Cheat Sheet
When choosing a data structure, it's crucial to understand how its performance scales with the size of the data. The time complexity refers to how the number of operations grows as the data size increases, while space complexity deals with how much memory is needed
Average case; worst case can degrade to O(n) with many collisions.
Key Principles for Arranging Data Efficiently
When working with data structures, one of the fundamental challenges is arranging data in a way that allows for quick and efficient access. For computer programmers and computer scientists, selecting the right data structure is crucial for performing functions like search, insertion, and deletion in optimal time. Each data structure serves a specific purpose based on how it stores and organizes all the elements within it.
For instance, hash tables map keys to values in a way that ensures fast lookups, making them ideal for tasks where quick retrieval of information is required. On the other hand, structures like trees or binary search trees are useful for hierarchical data, with each tree node representing a data element that connects to other nodes, forming a tree-like structure. This makes them ideal for applications where relationships between data points need to be represented efficiently.
When deciding on the next data structure to use in your project, it’s important to consider the underlying primitive data types (such as integers, floats, and characters) that will make up your data. For example, arrays are great for storing collections of simple primitive data types, while hash tables excel when dealing with more complex datasets that require fast lookups based on unique keys.
Understanding how to arrange data efficiently using these structures is key to optimizing your software's performance, allowing you to handle large volumes of data without sacrificing speed or memory efficiency.
Real-World Applications of Common Data Structures
Here are some examples of how common data structures are applied in the real world:
- Dynamic Programming: Arrays are used to store results of sub-problems, like in matrix chain multiplication.
- Job Scheduling: Heaps (priority queues) are used to select the highest-priority task.
- Caching Layer: Hash tables allow for fast data retrieval in web servers.
- Social Graphs: Graphs represent friendships and connections in social networks.
- Change Data Capture (CDC): Linked lists or logs track ordered changes.
- Autocomplete: Tries enable rapid prefix searches for search engines and applications.
How to Decide Which Data Structure to Use
Choosing the right data structure for your project can be daunting, but here’s a simple framework to guide your decision:
- Understand Your Data: Is your data hierarchical (tree-like) or flat (sequential)?
- Identify Dominant Operations: Do you frequently insert data? Use a linked list. Need fast lookups? Consider hash tables.
- Evaluate Time vs. Space: Arrays maximize cache locality, while hash data structures may use more memory for faster access.
- Consider Project Growth: Will the data structure handle increased data volume as your project scales?
- Leverage Ecosystem Libraries: Most programming languages have optimized collections—take advantage of them!
Tip: Profile early! A small O(n²) routine can drastically reduce performance when scaling to large datasets.

Unlock the Power of Data Structures with Airbyte
Modern data pipelines rely on a combination of data structures. Airbyte’s open-source platform uses queues for extraction scheduling, arrays for record batching, and hash tables for schema mapping—helping you synchronize databases, SaaS apps, and files with 600+ pre-built connectors. From arrays to graphs, understanding data structures is fundamental to building optimized software systems.
Each structure, whether linear, nonlinear, hash-based, or specialized, has its own advantages and trade-offs in terms of performance, memory usage, and scalability. Mastering data structures will allow you to solve problems more efficiently, making your software faster, more reliable, and scalable.
By understanding when and how to use each type of data structure, you'll be able to tackle complex software challenges and enhance the performance of your applications.
Explore supported data types and data structures in Airbyte here.