How to Use API in Python with Example (Python API Tutorial)
Python is a robust programming language used by developers to interact with different APIs. It provides advanced functionalities, allowing you to extract raw data from various applications and perform complex transformations to generate actionable insights.
Acting on these insights can be beneficial in improving business performance and enhancing customer experience. But how does one use Python to interact with APIs?
This article describes how to use API in Python and its advantages, with practical examples of extracting data using APIs to build data pipelines.
What Is an API?

Application Programming Interface, or API, is a set of rules and regulations that highlight how different applications communicate with each other. Working as an intermediary state, an API facilitates the interactions between client and server.
Here, the client initiates a request, and the server delivers an appropriate response to the given request. By bridging the gap between the client and the server application, APIs streamline the exchange of information, increasing interoperability across applications.
API HTTP Methods

API HTTP methods are crucial elements for sending requests and receiving responses. Each HTTP request has a unique name and functionality associated with it that enables the transfer of information between different systems. Some of the most commonly used HTTP methods include GET, POST, PUT, and DELETE.
API Status Codes

Whenever a request is made to an API, it responds with a status code indicating the outcome of the client request. The status code is an integral part of every HTTP request that represents whether the request sent to the server was successful or not.
Some of the standard status codes associated with the GET request include 200, 301, 400, 401, 403, 404, and 503. The codes starting with ‘4’ outline client-side errors, whereas the code beginning with ‘5’ indicates server-side errors.
API Endpoints
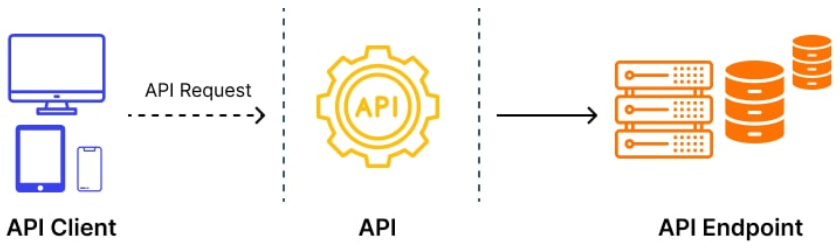
APIs allow access to specific data through multiple endpoints, which represent addresses that correspond to specific functionality. Executing an HTTP method with the API endpoint details enables access to the data available at a particular location. When working with APIs, some endpoints might just require address parameters, while others might require input fields.
Query Parameters
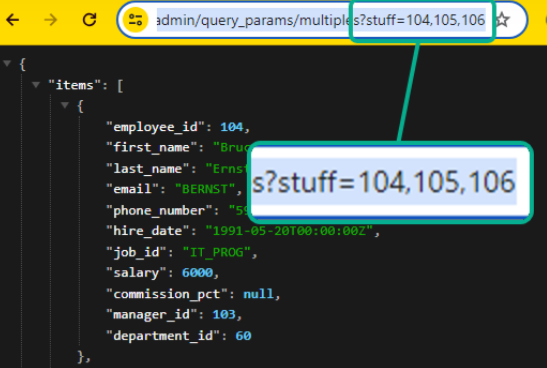
Query parameters are the most commonly used parameter types, which filter and customize the data returned by an API. It appears at the end of the URL address of the API endpoint.
For example, https://airline.server.test/ticket_api/{f_id}/{p_id}?option=business&option=vegeterian represents an address of an airline API. In this example,
- option=business&option=vegeterian are the query parameters.
- Outlining the query parameters restricts the data to specific values depending on the requirements.
Why Use API with Python?
Before getting started with how to use API in Python, you must first understand the why. Here are a few reasons demonstrating why working with APIs in Python is beneficial:
- Data Accessibility: One of the primary applications of leveraging Python for data engineering is extracting data from various sources and services. Python provides multiple libraries, including requests, urllib, and httpx to work with APIs, enabling you to pull data from different web applications.
- Flexible Data Storage: Python data types enable you to store the data effectively when working on an analytics workload. Based on data characteristics and operations to be performed on it, you can select the specific data type to store the collected API data. Allocating data to specific storages facilitates faster data retrieval and processing.
- Advanced Analytics: Using API with Python allows the execution of advanced analytics on the data received from the API request. By analyzing this data, you can produce practical business insights. Python provides robust libraries like scikit-learn and TensorFlow that can aid in creating powerful machine-learning models to answer complex questions.
- Parallel Processing: For use cases that require you to handle large sums of data, you can leverage Python’s multiprocessing module. This module lets you use multiple CPU cores to process tasks, optimizing performance while performing challenging computations.
How to Use API with Python?
Following a structured approach helps you effectively utilize Python with APIs to handle data from numerous sources. Let’s explore the steps illustrating how to use APIs in Python.
Install Required Libraries
Python offers several libraries for making API requests. To compare the libraries among the available options, you can refer to the points below:
- Requests: Preferable for simplicity and readability of the code and supports synchronous requests. It is ideal for use cases that require blocking of I/O operations.
- HTTPx: Supports both synchronous and asynchronous requests. It is a great choice for high-performance applications.
- Urllib: Part of the standard Python library. However, it is less intuitive and requires more code to perform straightforward tasks.
You can select any of the options mentioned and install it in a terminal. Use the pip install command to get the library:
Import it into a Python development environment.
Understanding the API Documentation
After importing the necessary library, it becomes crucial to understand the API documentation to utilize it effectively for your application. You must thoroughly review the guidelines for working with specific APIs. The API documentation provides information about different query parameters that you can use to access particular data.
Setting up Authentication
Working with the API of a platform requires authentication using specific credentials that aid in accessing data. To access information using an API, you must have the API key, which is a digital passcode that lets you access data from a specific source. By mentioning the page URL with the API key, you can retrieve data from any webpage.
For example, to access data from a website, ‘xyzapi,’ replace and specify the page URL with the API key.
Instead of hardcoding the API keys, it is considered beneficial to store them in environment variables. This step ensures the security of sensitive data from unauthorized access.
Making the API Request
Using the mentioned API key and URL allows you to perform API requests to access the web page content. Let’s execute a simple GET request to get the status of the connection:
Running the above code generates a response highlighting the status code of the connection setup. The output of ‘200’ signifies that the request was successful, and you can perform other operations. However, if the response is something else, you must refer to the API documentation and verify whether the URL or API Key has some issues.
Handling JSON Responses
APIs primarily exchange data in JSON—JavaScript Object Notation—format. In Python, JSON could be considered as a nested dictionary data structure that stores data in key-value pairs. To efficiently handle JSON data, Python offers a json library. This library provides two methods, including dumps() and loads().
The json.dumps() allows you to convert Python objects into JSON strings, whereas json.loads() facilitates the conversion of JSON strings to Python objects. For example, let’s use the JSON method to create a function for fetching and printing the data.
By providing the API endpoint to this function, you can get the specific content of the pages based on your requirements.
Example: Using News API in Python with Airbyte
Although working with APIs utilizing the Python libraries is an efficient method, there are certain limitations associated with this process. This method requires you to have prior technical expertise to manage issues that might arise when working with APIs.
Minor mistakes might result in errors that can be time-consuming to resolve. To overcome this challenge, you can leverage SaaS tools like Airbyte that enable you to interact with APIs almost effortlessly.
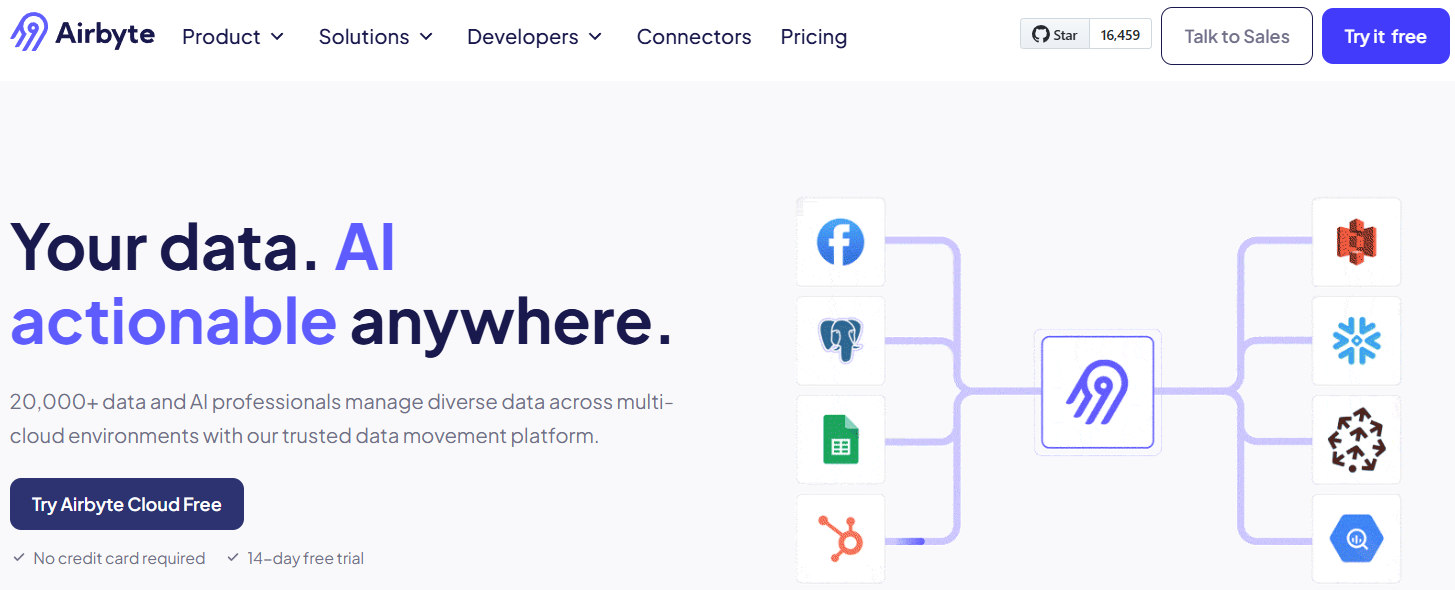
Airbyte is a no-code data integration platform that encourages you to migrate data from various sources to the destination of your choice. With more than 550 pre-built connectors, it helps you to directly interact with numerous tools and move structured, semi-structured, and unstructured data between them. If the connector you seek is unavailable, Airbyte provides Connector Development Kits (CDKs) and a Connector Builder to develop custom connectors.
Let’s go through some of the key features Airbyte offers:
- AI-Powered Connector Builder: The Connector Builder comes with an AI assistant that reads through your preferred connector’s API documentation and auto-fills most configuration fields. This feature makes the process of building custom connectors seamless.
- Change Data Capture (CDC): Airbyte CDC lets you automatically identify incremental changes made to the source data and replicate them to the destination file. This way, you can keep track of updates and maintain data consistency across different platforms.
- Vector Databases: Airbyte supports popular vector databases, such as Pinecone, Milvus, and Weaviate. By storing vector embeddings in these databases, you can train and build robust AI applications.
- RAG Techniques: You can integrate Airbyte with prominent LLM frameworks like LangChain and LlamaIndex to perform complex transformations, including chunking, embedding, and indexing, to streamline LLM response.
Along with these features, Airbyte offers a powerful open-source Python library, PyAirbyte, to build ETL pipelines in a development environment using Airbyte connectors. PyAirbyte eliminates the need to manually create the code or logic required to establish connections. Instead, you can configure the provided connectors to suit your specific data integration needs, making the process faster and more reliable. With this library, you can extract data from numerous data stores and APIs and load it into SQL caches. These caches are compatible with Python libraries like Pandas and LLM frameworks like LangChain.
Let’s explore how you can leverage PyAirbyte to interact with PokéAPI—A RESTful Pokémon API—to build flexible data pipelines. This API contains information about all the Pokémons with different attributes, including moves, abilities, and types. To extract data from PokéAPI using PyAirbyte, follow these steps:
- For the first step, install the PyAirbyte library on your machine. Open up your terminal and execute the code below:
- Open a code editor. Import PyAirbyte and configure the PokéAPI source connector. In this example, let’s set up a connection that will provide you with information about a specific Pokémon, Bulbasaur.
- To verify the source configuration details, execute the code below:
- You can now review the total number of data streams available for extraction in this API. Execute this code:
- If you require all the data streams for processing, run the following:
- Now, read this data stream into an SQL cache for temporary storage.
- Finally, convert this cache into a Pandas DataFrame.
After storing the data in Pandas DataFrame, you can perform complex transformations on the Pokémon data. Depending on your specific use case, you can either visualize this data or load it into a data warehouse for further analytics. For an in-depth tutorial, follow the Airbyte article on PokéAPI Python Pipeline creation.
How to Store Response Data from Python API?
When discussing how to use an API in Python, it is essential to understand the process of storing response data extracted using an API. API responses are often in JSON format, but they can be stored in CSV or other formats based on your needs.
To store the JSON response data in a file on your local machine, you can execute this code:
The above code snippet will create a file named ‘data.json’ in the same working directory.
If you prefer to store the API response in a CSV format, you can use Python’s built-in csv library. By creating a writer with the .writer() method, you can extract the data into a CSV file.
Conclusion
This guide comprehensively discusses how to use API in Python. By implementing a series of steps, you can extract data from any application and analyze the data to create effective business strategies. To simplify interactions with APIs, you can use tools like Airbyte for building efficient data pipelines.