How to Use JSON to Get Data from a Database: A Comprehensive Guide
All organizations depend on data-driven business strategies and require efficient data management for better analysis and decision-making. One way to handle your organizational datasets, especially during database operations, is to use a popular text-based format known as JSON (JavaScript Object Notation).
JSON data can be organized into key-value pairs, making it both human-readable and machine-parsable. Unlike relational databases, which require multiple tables, JSON enables complex data storage in a single, nested document. This structure helps you efficiently extract and manage heterogeneous data from various sources within JSON files.
Some key benefits of using JSON to get data from databases include its flexibility in representing varying data structures and compatibility with different programming languages. These advantages allow your business to improve data accessibility, streamline data processing, and accelerate database operations.
If you are eager to learn how to use JSON to get data from a database, this article has got you covered.
Understanding Database JSON Capabilities
Many database systems support JSON natively or through JSON-like structures due to its ease of use and flexibility in handling diverse data types. Relational databases, such as PostgreSQL, MySQL, and SQL Server, support native JSON data types to store JSON data directly within tables. This enables you to query JSON documents using specific JSON functions.
In contrast, databases that do not have native JSON types might use JSON-like structures, where the data is stored as text blobs. NoSQL databases like MongoDB are designed with JSON-like structures known as BSON (Binary JSON) for data storage with flexible schemas.
The ultimate difference is that native JSON types are stored directly within databases, which makes data handling faster without additional processing. On the other hand, JSON-like structures may need additional parsing to convert the data into usable format, which slows down the data management. Understanding this difference helps you decide on a database based on how you plan to work with JSON documents.
Preparing Your Database for JSON Extraction
To work with JSON data efficiently, you will need a database system that enables JSON functions. Some of the databases that support JSON functionalities include SQL Server, PostgreSQL, or MySQL. Once you select a database for JSON extraction, the next step is to utilize JSON operations for data management. If your data is scattered across various sources like APIs, CRMs, ERPs, or SaaS applications, you must first integrate it into the chosen database.
An easy way to do this is with Airbyte, an AI-powered data movement platform. It offers a user-friendly interface and 400+ built-in connectors to help you migrate data from any source to a destination of your choice. If you cannot find an Airbyte connector that fits your needs, you can easily create one in minutes with its no-code connector builder.
The latest Airbyte version, Airbyte 1.0, now offers an AI assistant in the connector builder to facilitate quick custom connector development. When you build a new source or destination connector, the AI assistant can help prefill and set up various fields during the configuration process.
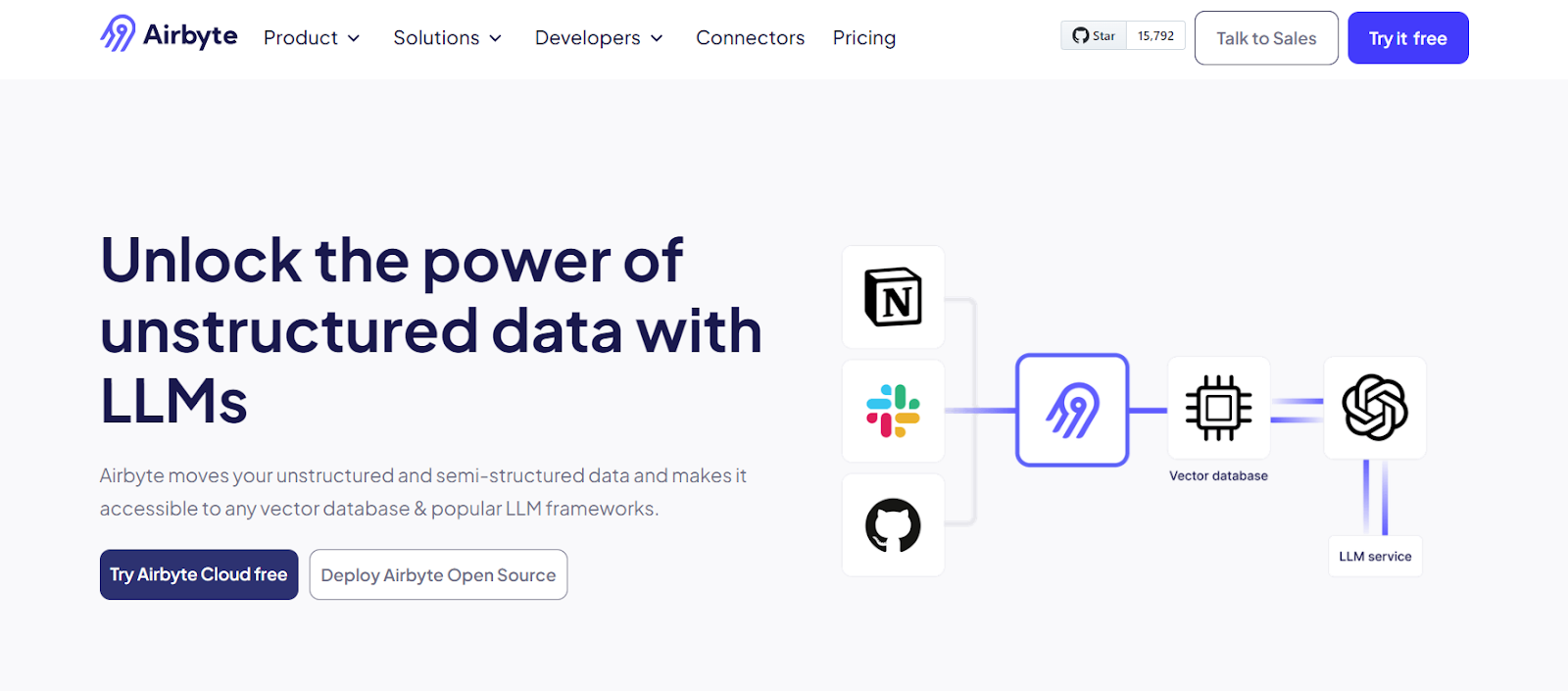
Let’s see how you can extract data from the database using Airbyte:
- Register or sign in to your Airbyte Cloud account. Alternatively, you can install Airbyte on your local system.
- Navigate to the left navigation pane of the Airbyte dashboard, choose the Sources tab, and search for your required source connector.
- Fill in the necessary fields to start configuring the source page.
- Click on the Set up source button.
- Go back to the left pane of the Airbyte dashboard, click Destinations, and search for your destination connector. For this example, let’s select MS SQL Server.
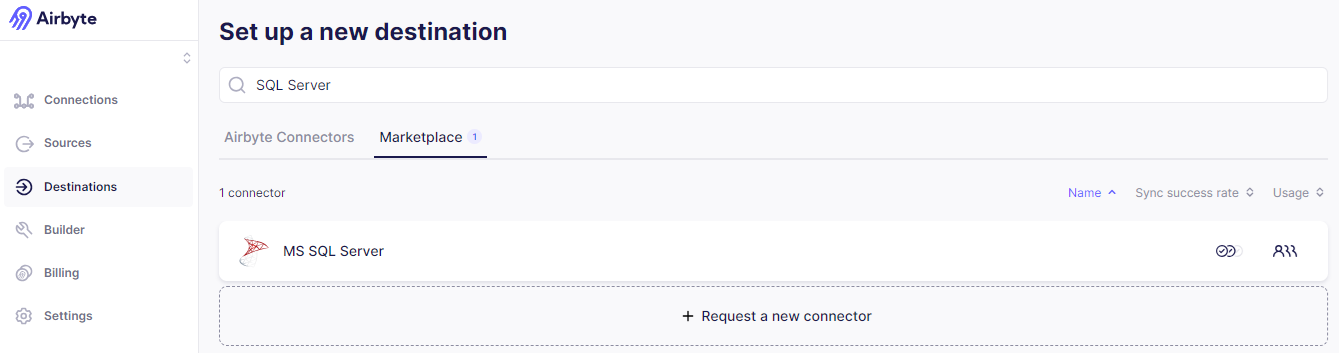
- You can now set up the SQL Server by referring to its setup guide on the right side of the configuration page.

- Once you specify all the required details, click the Setup Destination button.
After setting the source and destination, you need to establish a data pipeline between your source and SQL Server as follows:
- Click on the Connections tab from the Airbyte dashboard and select the + New Connection.
- Choose a recently configured source connector and SQL Server connector.
- Specify Sync frequency to set how frequently you want your data syncs to occur depending on your requirements.
- Select the data you want to import into the SQL Server as JSON. You can either sync all data or choose specific tables and fields.
- For your data streams, choose any one of the available sync modes: resumable full refresh, full refresh, or incremental syncs.
- Click the Test Connection button to ensure that your configuration works.
- Once the test passes, click the Setup Connection.
Following the above steps in Airbyte, you can transfer data from your chosen source system to the SQL Server. Similarly, you can migrate data from any other sources to JSON files or other JSON-enabled databases with Airbyte.
To extract JSON data from databases, you must query it using built-in JSON functions and operators. You can even configure the SQL Server settings to enable in-memory OLTP for optimizing JSON handling.
Basic JSON Queries
Once your data is loaded into SQL Server using Airbyte, you can start extracting data as JSON. Here is an example to understand how basic JSON queries are performed in SQL Server:
Consider a table named “Sample_Table” with the attributes “id,” “firstName,” “lastName,” and “age” within an SQL Server database instance.
In SQL Server, the FOR JSON clause is used in the SELECT statement to return query results in JSON format.

The query result set would be:
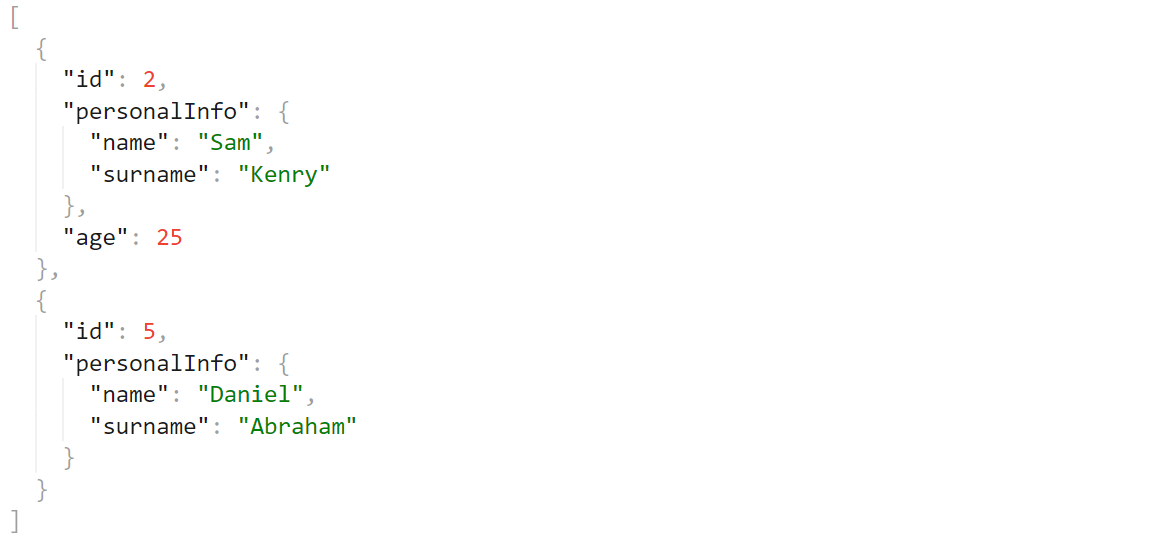
You can extract, filter, and manipulate JSON data in SQL Server using its built-in JSON functions as follows:
- JSON_VALUE: It helps you retrieve a scalar value from a JSON string.
- JSON_QUERY: It allows you to extract an array or object from a JSON string.
- JSON_MODIFY: It enables you to update the property value in a JSON string and returns the modified one.
- ISJSON: It checks whether a string contains valid JSON.
Along with these functions, you can use mathematical, string, and comparison operators in a query to transform the JSON data.
If you transfer your data to an object-relational database like PostgreSQL, you will find the pre-defined JSON functions, including:
- row_to_json(): Converts rows into JSON objects.
- to_json(): Transforms values into JSON.
- json_agg(): Aggregate rows into a JSON array.
PostgreSQL also offers JSON-specific operators, such as ->, ->>, #>, and #>>, to retrieve JSON array elements or object fields.
Similarly, many other popular databases also support JSON functions and operators to query and transform the JSON data efficiently.
Advanced JSON Extraction Techniques
Here are the two advanced JSON extraction techniques supported by various programming languages:
JSONata
JSONata is a lightweight query and transformation language that you can implement in Python, Go, Java, and Rust to work with JSON data. It allows you to query, transform, and extract relevant information from JSON structures without complex code. Like XPath, a language to query XML data, JSONata adopts location path syntax to perform basic to complex queries. This enables you to navigate through a deeply nested structure of JSON objects using dot (.) notation to select the required data from the JSON document.
For example,

To select the Animal’s name, you would query Animal.Name, which would result in “Dog.” In this way, you can access any data in a JSON object.
In addition, JSONata supports a rich set of functions and operators. You can use mathematical operators like +, -, *, and / to perform calculations and string functions such as $length() or $substring() for string manipulation. Aggregation functions such as $sum(), $max, $min, and $average help you work with arrays.
With JSONata, you can also combine queries, functions, and operators efficiently. Let’s modify the above JSON structure with an array of the same animal objects and a new field called “Age.”

Here is the JSONata query to extract the sum of the ages of German Shepherd dogs older than two years:
The output of the query is 9.
This example shows JSONata as a versatile tool for querying and processing JSON data.
JMESPath
JMESPath is a powerful query language designed for JSON data. It provides a set of expressions to navigate through nested JSON structures and handle arrays within it. You can implement JMESPath in Python, JavaScript, Ruby, or Go.
Considering the above example, let’s illustrate how JMESPath helps to extract a field:
Animals[*].Age
This query will output [4,1,5]
Transforming Relational Data to JSON
You can transform the relational data into JSON by converting rows to JSON objects, grouping related data into nested JSON structures, and handling one-to-many relationships. Let’s see how to achieve these transformations:
- Converting Rows to JSON Objects: Data in a relational database is organized into tables with rows and columns. Each row can be represented as a JSON object, where the column names become the keys, and the corresponding values in the row are the values. Functions like row_to_json() help you perform this conversion in PostgreSQL.
- Grouping Related Data into Nested JSON Structures: Like connecting two or more relational tables, you can group the associated data into nested JSON structures. This is useful for representing hierarchical relationships within the data.
- Handling One-to-Many Relationships in JSON: Once you group data from multiple tables into nested JSON structures, you must maintain the relationships between the JSON objects clearly. It indicates that you should include all related child objects in a way that shows their association with each parent object.
Optimizing JSON Extraction Performance
To optimize JSON extraction performance, you can use path-based, function-based, or partial indexes on frequently queried JSON objects, which speeds up data retrieval. To reduce the amount of data processed during query execution, you can use the LIMIT clause or select only the necessary fields from the JSON objects. Additionally, analyzing query execution plans can help identify bottlenecks and modify your queries accordingly.
Currently, modern databases support hybrid data models that combine JSON with traditional structures, all accessible through standard SQL. For new or refactored applications, adopting a hybrid relational/JSON model facilitates enhanced development efficiency while providing the flexibility to adapt to future needs.
Error Handling and Data Validation
Effective error handling and data validation are essential when working with JSON, especially in complex data environments. If JSON data is malformed because of parsing errors, schema mismatches, or data loss during migration, the data processing flow will be affected. This will prevent the applications from interpreting the data correctly to produce accurate results. To handle this, implement error-catching mechanisms that detect and log invalid JSON strings.
Libraries like Python’s json module or Javascript’s JSON.parse() often have built-in methods for catching these errors and taking corrective action. Tools like jsonschema in Python or Ajv in JavaScript help you to validate against a defined schema during extraction. It ensures that required fields, data types, and formats are correct before the JSON is processed further.
Apart from these, JSON data can often include NULL values or missing certain keys. Using default values for missing keys or conditional checks to manage null entries will allow you to manage incomplete or partial JSON data.
Working with Large Datasets
When working with large datasets in JSON format, it is important to optimize data trivial and processing. Streaming JSON results allows you to manage memory efficiently by transmitting data in chunks rather than loading the entire dataset simultaneously.
Pagination techniques can be used to enhance performance further. They enable you to break large data into smaller, more manageable units, helping you process data page by page.
Along with these approaches, you can apply chunking strategies that allow you to divide the data by character, sentence, or semantics into small partitions. This ensures that memory usage remains stable and processing remains efficient even with extensive datasets.
Cross-Database JSON Operations
Cross-database JSON operations involve extracting JSON data from one database to another. During this process, you must handle the format differences between the databases, as each system may store or interpret JSON differently. As a result, it is necessary to ensure that the data remains consistent and correct to maintain data integrity across systems. This will prevent any loss or corruption of the JSON data during the transfer.
API Integration for JSON Extraction
You can create REST APIs using database connectors like SQLAlchemy, Connexion, and Flask to get data from databases in JSON format. Implementing GraphQL, a query language for your API, can help with more flexible querying. It enables you to request the data you need in JSON format. Securing JSON-based APIs is crucial to protecting this data; only authorized users can access or modify sensitive information.
Real-Time JSON Data Extraction
You can use the Change Data Capture (CDC) approach to track changes in a database and output them as JSON. This helps you stream JSON updates directly from the database, making it possible to process the changes instantly. Applications can then handle this real-time JSON data, ensuring they stay up-to-date with the latest information and respond quickly to changes without delays.
Summary
You have explored how to use JSON to get data from a database. Using JSON to get data from databases improves your ability to manage and utilize data effectively. Its flexibility, compatibility with various database systems, and support for complex data structures make it a suitable choice for modern applications.
By understanding your database's JSON capabilities, preparing it for JSON extraction, and using advanced querying techniques, you can streamline your data operations. Optimizing JSON extraction performance, ensuring robust error handling, and implementing real-time data streaming further enhance your workflow.