Database Triggers in SQL: How to Use & Create Triggers
Maintaining data integrity, tracking updates, and enforcing business rules on data are essential for effective database management. However, manually performing these operations can be challenging and time-consuming. To simplify such complex tasks and automate repetitive operations, you can use database triggers in SQL.
Database triggers are valuable components that allow you to execute multiple tasks based on certain events. This guide will comprehensively cover database triggers in SQL and how you can use them to automate your data workflow.
What Are SQL Database Triggers?
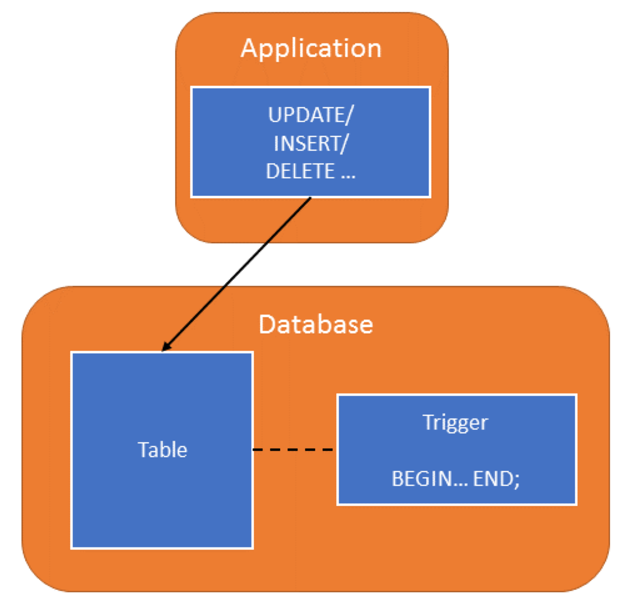
Database triggers in SQL are special objects that store executable logic, automatically triggered by certain database events. Often linked to particular tables, these triggers consist of a series of SQL statements that are stored in system memory. If the associated table is dropped, the corresponding triggers are also removed.
Triggers are an essential component of advanced SQL concepts that offer you the capabilities to simplify complex, repetitive tasks. The triggers are invoked before or after INSERT, UPDATE, and DELETE operations on a given table. During the execution of these commands, triggers are automatically invoked to perform predefined actions.
Triggers vs. Stored Procedures
Types of Database Triggers in SQL

Database triggers in SQL are of different types based on their associated actions/tasks. Let’s discuss each type in detail.
Data Manipulation Language (DML) Triggers
DML triggers activate in response to DML commands, such as INSERT, UPDATE, and DELETE statements. You can define these triggers to execute AFTER, BEFORE, or INSTEAD OF the operations. This allows the triggers to modify or validate the data changes before they’re committed to the table or view.
Data Definition Language (DDL) Triggers
DDL triggers are triggered in response to DDL events, such as CREATE, ALTER, and DROP statements. These triggers are especially useful for applications that require managing schema changes, auditing modifications to the database structure, and enforcing security standards.
Logon Triggers
Logon database triggers in SQL are executed during LOGON events. The triggers are useful for monitoring login activities and managing server sessions, such as restricting the number of concurrent sessions a user can have. To specify a logon trigger, use the CREATE TRIGGER trigger_name ON LOGON command.
BEFORE/AFTER Triggers
Before/After triggers use BEFORE and AFTER keywords to define the time of execution of any query. For example, if you use a before trigger to execute some task, you must specify the BEFORE keyword with the associated statement, BEFORE UPDATE ON. This statement performs operations before the execution of any update on the table.
Row-Level Triggers
Row-level triggers operate on each row affected by the triggering event. These triggers are beneficial for maintaining data integrity and enforcing business logic on the table. An example is when you define a row-level trigger with the INSERT command. When new rows are added to the table, the row-level trigger automatically executes for each new row. The same holds for each row that you update or delete.
Statement-Level Triggers
Statement-level database triggers in SQL represent the overall effect of different DML commands in a single operation. Unlike row-level triggers, these triggers execute only once per triggering SQL statement, regardless of how many rows are affected. A statement-level trigger is an effective choice for triggering notifications for bulk changes.
How to Create Your First Database Trigger in SQL?
There are multiple ways to create database triggers in SQL. Although the syntax for creating triggers changes from one database to another, the basic underlying logic remains the same.
To define triggers in MySQL, you can follow this syntax:
In the above code:
- The trigger_name is the name of the trigger you are creating.
- trigger_time specifies when the trigger should execute. It can be set to AFTER or BEFORE the event.
- trigger_event mentions the event that activates the trigger. It can be INSERT, UPDATE, or DELETE.
- table_name is the name of the table where the logic will be applied.
- trigger_order contains the order of execution if multiple triggers exist for the same event on the table.
- trigger_body is the SQL code that is to be executed after the invocation of a trigger.
SQL Database Trigger Examples
Database triggers in SQL have a wide range of benefits in real-world applications. This section gives you an overview of some of the most crucial use cases.
Audit Trails
Using database triggers enables you to track database events like data entries, updates, deletes, and access attempts. This allows you to maintain accountability and transparency by recording important actions within your database.
For example, you can set up a trigger to capture data updates in a specific table by running the code below:
The above code tracks the critical changes by logging the updates, including the old and new values associated with the data row. This trigger is invoked before the execution of the UPDATE statement.
Enforcing Business Rules
With database triggers, you can enforce business rules directly within the database. This helps ensure that your data adheres to predefined rules. For example, you can ensure any product’s price is always at least 5% higher than its original price to maintain a profit margin.
Maintaining Database Integrity
Database triggers are crucial for maintaining data consistency and integrity. You can consider an example where you define a trigger to ensure data consistency. The trigger will replicate a foreign key in the summary table after the insertion of a new record into the database.
Best Practices to Follow When Creating SQL Database Triggers
Following certain best practices when creating SQL database triggers can optimize database performance and improve database management. Here’s a list of best practices to follow:
- Simplify Trigger Logic: Keep the SQL logic within triggers simple to avoid performance issues. By minimizing complex transformations and operations in triggers, you can reduce latency and ensure effective execution.
- Trade-offs: Consider all the benefits and drawbacks of implementing database triggers in SQL. Assess whether the automation and integrity enforced by the trigger justify the processing overhead and additional complexity.
- Documentation: Document all the necessary details, including the trigger's operations, its purpose, and additional implementation information. This will help you with maintenance and troubleshooting, especially as systems scale.
Limitations of Database Triggers in SQL & How to Avoid Them
While database triggers provide you with robust automation capabilities, they have some limitations. Let’s look into the common drawbacks of database triggers and how you can avoid them.
- Complexity: In some instances, creating database triggers from scratch can be quite challenging, especially for complex business logic. To overcome this issue, you must break down the logic into smaller, more manageable components. Develop each part incrementally to ensure clarity and for easier maintenance.
- Restrictions in Validation: While triggers can handle complex validation scenarios, simple validation tasks are better managed by SQL constraints. For example, NOT NULL, UNIQUE, FOREIGN KEY, and CHECK constraints provide a simple alternative to creating triggers.
- Performance Overhead: Database triggers can significantly increase overhead as they execute automatically in response to events. This issue might degrade database performance. Alternatives like stored procedures and user-defined functions are better choices. You can execute these when needed rather than automatically, reducing unnecessary performance impacts.
Are There Any Alternatives to Database Triggers?
There are several alternatives that can help achieve similar outcomes as database triggers, but with fewer drawbacks:
- Temporal Tables: Introduced in SQL Server, temporal tables offer an easier way to add versioning to tables. They include a history table that automatically stores previous data versions.
- Functions: Functions are reusable database objects that consolidate SQL logic, which you can apply to data as required. You can use a single function for multiple tables, performing the same logic as triggers but more efficiently.
- Stored Procedures: Replacing triggers with stored procedures provides you the flexibility to structure tasks and adjust atomicity as needed. Implementing logic with stored procedures helps reduce the complexity, contention, and obscurity that might come with database triggers.
- Check Constraint: For simple data validation tasks, defining a check constraint could be a better alternative to triggers. You can create a check constraint on a column to automatically validate data upon entry. For example, you can check whether the new data is in a specified format. If it isn’t, the check constraint displays an error on the terminal.
- Unique Constraint: By adding a unique constraint to your table, you can ensure that the data entries are not duplicated. If the new data is a copy, the insertion will automatically fail, preventing duplicate data from being in the table.
- Foreign Key Constraint: Similar to check and unique constraints, configuring foreign key constraints is another way to validate data integrity. This constraint checks whether the data in the foreign key column matches existing values in the reference table. If it doesn’t, the operation is eliminated, and an error message is displayed.
Utilizing Airbyte to Streamline Database Triggers Operations
Inserting data from any business-centric tool into a database is an essential step in workflows that operate ETL and SQL simultaneously. By migrating data into your database, you can apply business logic using triggers. However, manually transferring data can be challenging and time-consuming. To resolve this complexity, you can use SaaS-based tools like Airbyte to streamline data migration.

Airbyte is a no-code data replication tool that allows you to transfer data from multiple sources to a destination of your choice. With over 550 pre-built connectors, it lets you migrate structured, semi-structured, and unstructured data into your preferred database. However, if the connector you seek is unavailable, Airbyte offers products like Connector Development Kits (CDKs) and a Connector Builder for developing custom connectors.
Let’s explore some of the most impressive features offered by Airbyte:
- AI-Powered Connector Builder: The Airbyte Connector Builder comes with an AI assistant that reads through your preferred platform’s API documentation and auto-fills most configuration fields. This functionality simplifies the connector development process for you.
- Change Data Capture (CDC): Utilizing the CDC feature, you can identify the incremental changes in the source data and replicate them to your database. This helps maintain consistency by monitoring data updates.
- Schema Management: The schema change management feature enables you to mention how Airbyte must handle source schema changes for each connection. For Cloud users, Airbyte automatically performs schema changes every 15 minutes, whereas for Self-managed accounts, the checks are performed at most every 24 hours.
Conclusion
Database triggers in SQL are objects that store SQL logic, which is executed when certain conditions are met. If the specified condition is triggered, the logic executes, performing predefined operations on your data.
Although using a trigger is beneficial to document changes in the database, you must also consider the disadvantages that come with them. In such cases, alternatives like stored procedures, functions, and constraints in some scenarios can reduce the overhead associated with triggers. By clearly understanding the situations where using a trigger would be beneficial, you can optimize business operations on the database.
💡Suggested Read: