Python For ETL: How to Build ETL Pipelines With Examples
Python is one of the most popular modern programming languages. Its easy syntax, extensive library ecosystem, and huge adaptability make it a go-to choice for data engineers worldwide, especially in data management. Most professionals use Python to do most of the data management tasks. One classic example of that is ETL. An ETL is a workflow that aims to take difficult-to-use or unstructured data and serve it to the source in a clean and structured format.
In this comprehensive article, you'll explore the entire process of setting up an ETL pipeline using Python.
What is ETL?
To understand Python ETL, let’s first understand the ETL. ETL or Extract, Transform, Load is a traditional way of integrating data between two or more operational systems. The process originated when on-premise servers and hardware-based solutions were used for managing data from disparate sources. Since then, ETL has evolved a lot, but the core concepts are still the same. It is a three-step process that synchronizes multiple data sources to centralized destinations such as analytical platforms or data warehouses.
An ETL pipeline consists of the following:
Extraction: In this phase, the data is pulled from multiple data sources such as third-party applications, APIs, flat files, excel sheets, or other transactional systems. This step aims to process and store the data from disparate sources in a staging area.
Transformation: Here, the raw data undergoes data processing to be structured according to the requirements of the destination. It can involve the following tasks: cleansing, aggregation, manipulation, validation, filtering, and formatting.
Loading: Lastly, the transformed data is moved from the staging area to the target destination.
Now, you can carry out this task in different ways, such as using automation tools like Airbyte, programming languages like Python, or a combination of both. The method of executing ETL using Python is called Python ETL.
So, in Python ETL, you are carrying out the entire process of extracting, transforming, and loading with Python programming language.
Benefits of Using Python for ETL Process
Let's dive into the advantages of using the Python ETL framework:
Vast Ecosystem of Libraries and Frameworks
Python's expansive ecosystem includes a wide array of libraries and frameworks specifically suited for ETL processes. These libraries make performing complex transformations on large datasets easier and more efficient.
For example, pandas and NumPy provide robust data manipulation capabilities, while SQLAlchemy enables seamless connectivity with various databases. On the other hand, Beautiful Soup is a great choice for web crawling and data extraction.
Integration Capabilities
Python is popular for its seamless integration capabilities with various data sources and destinations. It supports a wide range of databases, file formats, and APIs, allowing for smooth data extraction from different sources.
Additionally, Python is compatible with big data tools like Apache Spark (PySpark), RESTful APIs (requests), and messaging systems such as RabbitMQ (pika). This highlights its versatility in integrating with various systems.
ETL Pipeline Flexibility
Python supports multiple paradigms and programming styles, such as object-oriented, functional, and procedural. This enables you to choose the best approach for designing and implementing your ETL logic.
For example, if your destination system involves cloud storage services like Amazon S3, boto3 is a powerful library that helps to load your data seamlessly.
Active Community of Data Scientists
Python has a large, supportive community of data scientists who actively contribute to the development and maintenance of libraries. Extensive documentation, tutorials, and forums are available to provide support and assistance. This empowers you to optimize your ETL workflows and stay up-to-date with the latest developments in data processing.
How to Build ETL Pipeline with Python?
Building an ETL pipeline using Python is a powerful way to efficiently manage data processing tasks. Here are the key steps to consider:
Define the Data Sources and Destinations
Identify the sources you need to extract data, such as databases, flat files, or APIs. Also, determine the target system where the transformed data will be loaded, such as data warehouses, databases, or data lakes.
Plan the Flow of Data from Source to Destination
Map out the entire data flow, including the sequence of transformations required to convert the raw data into the desired format for the destination. This step helps you understand the dependencies and design the pipeline effectively.
Set Up the Development Environment
To build the ETL pipeline, you'll need to set up a Python development environment. This typically involves installing Python and any necessary libraries or frameworks based on your requirements. Popular libraries for ETL tasks include pandas, NumPy, and SQLAlchemy.
Extract Data from Sources
To extract data from various sources, Python offers a range of powerful libraries tailored to different data retrieval needs. For databases, SQLAlchemy can be used to establish connections, execute queries, and retrieve data efficiently. When working with APIs, you can use the requests library, as it simplifies the process of making API requests and handling responses.
Transform the Data
Apply any necessary transformations to the extracted data. This might include cleaning the data, filtering rows, joining tables, or performing calculations. Utilize libraries like pandas to manipulate the data effectively.
Load the Data into Destinations
Once the data is cleansed and transformed, load it into the specified destinations, such as a database or data warehouse. Python offers a variety of robust libraries and interfaces that make this process efficient and seamless. For instance, with dlt (data load tool), an open-source library, you can easily load data into your destination system.
Schedule the ETL Pipeline
To ensure the ETL pipeline runs regularly, you'll need to set up a scheduling mechanism. You can leverage Python modules like crontab or platforms like Apache Airflow for more advanced workflow management.
Implement Error Handling and Logging
Handle exceptions that may occur during the ETL process. Ensure that errors are logged and handled properly to prevent data loss and ensure pipeline reliability. You can use Python's 'logging' module to keep a detailed log of pipeline execution.
Deploy and Monitor the ETL Pipeline
Deploy your ETL pipeline to the production environment and set up monitoring to ensure its smooth operation. Monitor data quality, performance, and any potential failures.
How to Set up Python ETL?
In this section, you will create a basic Python ETL framework for a data pipeline. The data pipeline will have essential elements to give you an idea about extracting, transforming, and loading the data from the data source to the destination of your choice. For the sake of simplicity, the data source will be CSV, and the destination will be MongoDB for a practical example. Here’s a detailed guide:
Prerequisites
- Python.
- A Windows PowerShell.
- A working MongoDB database.
Step 1: Install & Import Required Packages
Importing the right libraries is the first step toward creating anything using Python. This step includes using two libraries to make an ETL pipeline: pandas and pymongo. To achieve this task, type the following code in the command line:
The pandas library can be used to transform and manipulate data, and the pymongo library helps interact with MongoDB in a Python project.
Step 2: Extracting Data from Source
The extraction process in Python varies based on the data source. A data source could include a database, flat file, CSV file, API, or an application. As mentioned above, this method involves extracting data from the CSV file in this ETL pipeline. Make a file named etl.py in your local machine and paste the code below to perform this task:
In the above code, the pandas library is imported, and the data from your_csv_file.csv is saved into the data variable.
Step 3: Transforming Data in Required Format
In this step, you will transform data in format and sequence according to your requirements. With Python’s modern syntax and pandas' data transformation functionalities like aggregation and manipulation, there is a lot of scope for enhancing datasets. Below are some examples of the basic transformations with CSV data using Python:
Sort and Filter
One common use case when transforming is ordering data. To do this, you can use methods like sort_values and filter from the pandas library. Here’s how to use these methods:
In the above code, a hypothetical example is given as a name in the sort_values method to sort the name field alphabetically from the data stored in the CSV file. However, in the filter method, you can provide specific columns in this example (name, is_student, target), and only those columns will appear in the results.
Removing Duplicates
A common challenge in raw datasets is duplicate rows of data. The code below demonstrates finding and removing duplicates using the drop_duplicate method of the pandas library:
Above are the two common examples of basic transformations of Python ETL. You can choose to perform more transformation practices according to your requirements.
Step 4: Loading Data in MongoDB
This is the most technical step of the ETL process. However, if you have a basic working knowledge of Python, you’ll be fine. Here’s how to load the CSV data to MongoDB with Python:
First, you must connect MongoDB to Python:
In the above code, replace your_database with the name of your MongoDB database and your_collection with the collection name.
To copy the CSV data to MongoDB, you must use the to_dict method of pandas and create JSON data (list of dictionaries) for inserting multiple records in MongoDB. Here’s the code:
Complete Code for Python ETL
Here is the complete code for the Python ETL pipeline you created. You can use Python IDE’s like Jupyter Notebook to execute this command:
Note: The above code does not involve transformation to the CSV file before loading, as it depends on your specific requirements.
That's it. If you carefully follow the steps mentioned above, you can create a basic Python ETL framework to migrate data from the CSV file to MongoDB.
Common Python Libraries Used in ETL Pipelines
Here is an overview of popular Python libraries in ETL pipeline development:
PyAirbyte
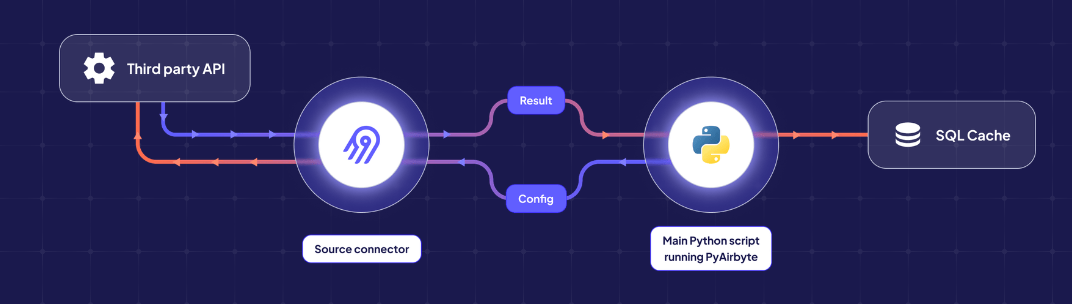
PyAirbyte is a robust Python library developed by Airbyte that allows you to easily build ETL pipelines. It facilitates automated data extraction and loading processes through Airbyte supported connectors, ensuring that data flows smoothly from source to destination. This helps maintain data integrity and consistency across the ETL pipeline.
Pandas

Pandas is a widely used Python library for data manipulation and analysis. You can handle missing data, remove duplicates, handle outliers, convert data types, and perform various transformations on the data. This helps to ensure that the data is in a suitable format for analysis.
SQLAlchemy

SQLAlchemy is a comprehensive Python SQL toolkit and Object Relational Mapper (ORM) that facilitates smooth interaction with databases. It allows you to map Python objects to database tables, simplifying the interaction with relational databases.
You can define classes that represent database tables, and SQLAlchemy handles the mapping between the objects. This enables you to perform database operations using Python code rather than writing raw SQL queries.
requests
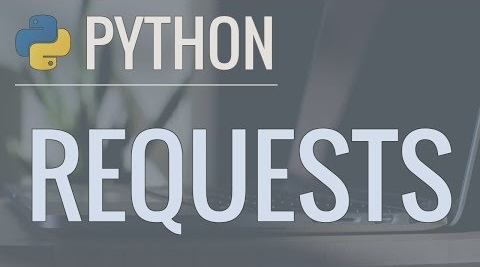
requests is a widely used Python library for making HTTP requests, which is crucial for collecting data from web services and RESTful APIs. It enables you to effortlessly send HTTP/1.1 requests without the hassle of manually appending query strings to your URLs. This streamlined approach facilitates easier integration with web services, making data extraction more efficient.
NumPy

NumPy is a fundamental library for numerical computing in Python, offering support for large multi-dimensional arrays and matrices. It is commonly used in ETL processes to efficiently perform complex data manipulations and transformations on large datasets.
Beautiful Soup
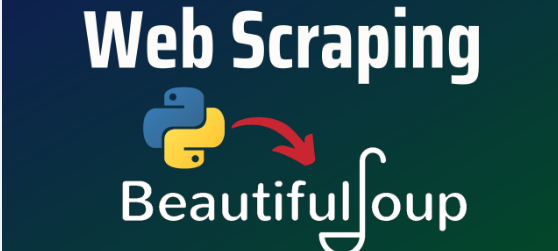
Beautiful Soup is a Python library designed for web scraping projects. It lets you parse and extract useful information from HTML and XML documents. This enables you to search for specific elements or patterns within the HTML document using various search methods. You can use various methods, such as find_all(), find(), select(), and more, to filter the data based on specific criteria.
Python ETL Use Cases & Examples
Let's explore some Python ETL examples in various domains:
Finance
Real-time data processing is essential in financial trading to make timely and informed decisions. For example, the 'confluent-kafka-python' library can be leveraged to analyze stock prices. It captures real-time market data from multiple sources, such as stock prices and trading volumes. This data can be streamed into Kafka topics, where it is immediately available for consumption by different downstream applications.
Social Media Data Collection
Social media platforms generate massive data that businesses can analyze to gain insights into customer sentiments and market trends. By leveraging libraries like Tweepy and Requests, data can be extracted from Twitter and transformed into a structured format for analysis.
E-Commerce
E-commerce businesses can develop Python ETL pipelines to consolidate and analyze customer data from various sources, such as purchase history, browsing history, and search queries. For example, Python libraries like pandas can be utilized to manipulate data, and scikit-learn can be used to build AI models. This will help you to identify customer purchase patterns and personalize marketing strategies.
Limitations of Using Python for ETL
While Python is a popular choice for ETL tasks due to its flexibility and the rich ecosystem of libraries, it does come with certain limitations. Here are a few of them:
Low Performance
Python is an interpreted language, which means it runs slower than compiled languages such as Java or C. This can impact the performance of ETL processes, especially when dealing with huge and complex data sets.
Memory-Intensive
Python's data structures, particularly Pandas DataFrames, can be quite memory-intensive. This can result in significant memory usage and potential memory overflow issues when dealing with large datasets.
Scalability
As data volumes and complexity increase, Python code can become extensive and more complex, making it harder to maintain. Additionally, Python's high memory consumption and limited concurrency due to the Global Interpreter Lock (GIL) can impact scalability.
Data Type Handling
Python can sometimes experience issues with data type handling, especially when interacting with various data sources and destinations. Different databases and file formats may have different data type standards, and Python's dynamic typing system may not always handle these disparities smoothly. This can lead to data inconsistencies and potential errors during the ETL process.
Error Handling
ETL processes often involve data from multiple sources and formats. Writing error-handling code that can effectively deal with all these variations is complex and time-consuming.
Technical Complexity
The example used to demonstrate Python ETL is very basic. In real-life conditions, the operations you must perform will be technically complex.
Expertise Requirement
As you might have seen above, with this much coding involved in the practice, any professional with a non-technical background can't execute the task. Therefore, a level of technical proficiency is required for Python ETL.
Time & Resource
Manually programming each workflow for ETL, whenever required, needs a dedicated team of developers for each specific task involved. This can be time-consuming and resource-intensive for your organization.
How can PyAirbyte Solve the Problems of Traditional ETL with Python?
Here's how PyAirbyte can efficiently address the challenges of traditional ETL using Python:
Complexity of ETL Scripts
Problem: Traditional ETL processes often require manual coding in pipeline development, which can be error-prone and require significant development time.
Solution: PyAirbyte reduces the need for custom ETL coding through a vast library of pre-built connectors. It allows you to effortlessly connect to various data sources like APIs, databases, and SaaS applications. This streamlined approach lets you quickly set up data pipelines and extract insights from your data.
Scalability
Problem: Scaling traditional ETL pipelines to handle growing data volumes can be challenging and often requires significant architectural changes.
Solution: PyAirbyte can handle large data volumes efficiently. It writes data to disk first and compresses it, ensuring fast and memory-efficient processing. Therefore, it is easier to manage increasingly complex data workflows.
Lack of Interoperability
Problem: Traditional ETL processes may face challenges when it comes to interoperability with other tools and frameworks.
Solution: PyAirbyte overcomes this problem by ensuring compatibility with various Python libraries, such as Pandas and SQL-based tools. You can also integrate seamlessly with popular AI frameworks, such as LangChain and LlamaIndex, to develop LLM-powered applications.
Conclusion
In this article, you have learned how to set up an ETL pipeline using Python, gaining a comprehensive understanding of the entire process. You have also discovered the significant benefits of the Python ETL framework, including its flexibility and extensive library support.
FAQ’s
Is Python good for ETL?
Yes, Python is well-suited for ETL tasks. It provides a wide range of libraries and frameworks that enable you to extract data from various sources easily, transform it as needed, and load it into different destinations.
Can we use pandas for ETL?
Yes, Pandas is widely used for ETL tasks. It provides robust data manipulation capabilities for cleaning, transforming, and analyzing data efficiently.
Should I use pandas or SQL for ETL?
The choice between pandas and SQL for ETL depends on the specific requirements of your project. If you are working with structured data and prefer a SQL-like approach, using SQL for ETL might be a good option. On the other hand, if you need more flexibility and advanced data manipulation, pandas can be a better choice.
Which IDE is best for ETL with Python?
There are several IDEs that are commonly used for ETL with Python, such as PyCharm, Jupyter Notebook, and Visual Studio Code. However, the best IDE depends on your personal preference and project requirements.
Python or SQL for ETL? Or both?
Both Python and SQL have their strengths for ETL. Python provides many libraries and tools for data manipulation and analysis, making it suitable for complex transformations. SQL, on the other hand, is well-suited for querying and manipulating structured data in databases. Depending on the task, you can use Python, SQL, or both to achieve your ETL goals.